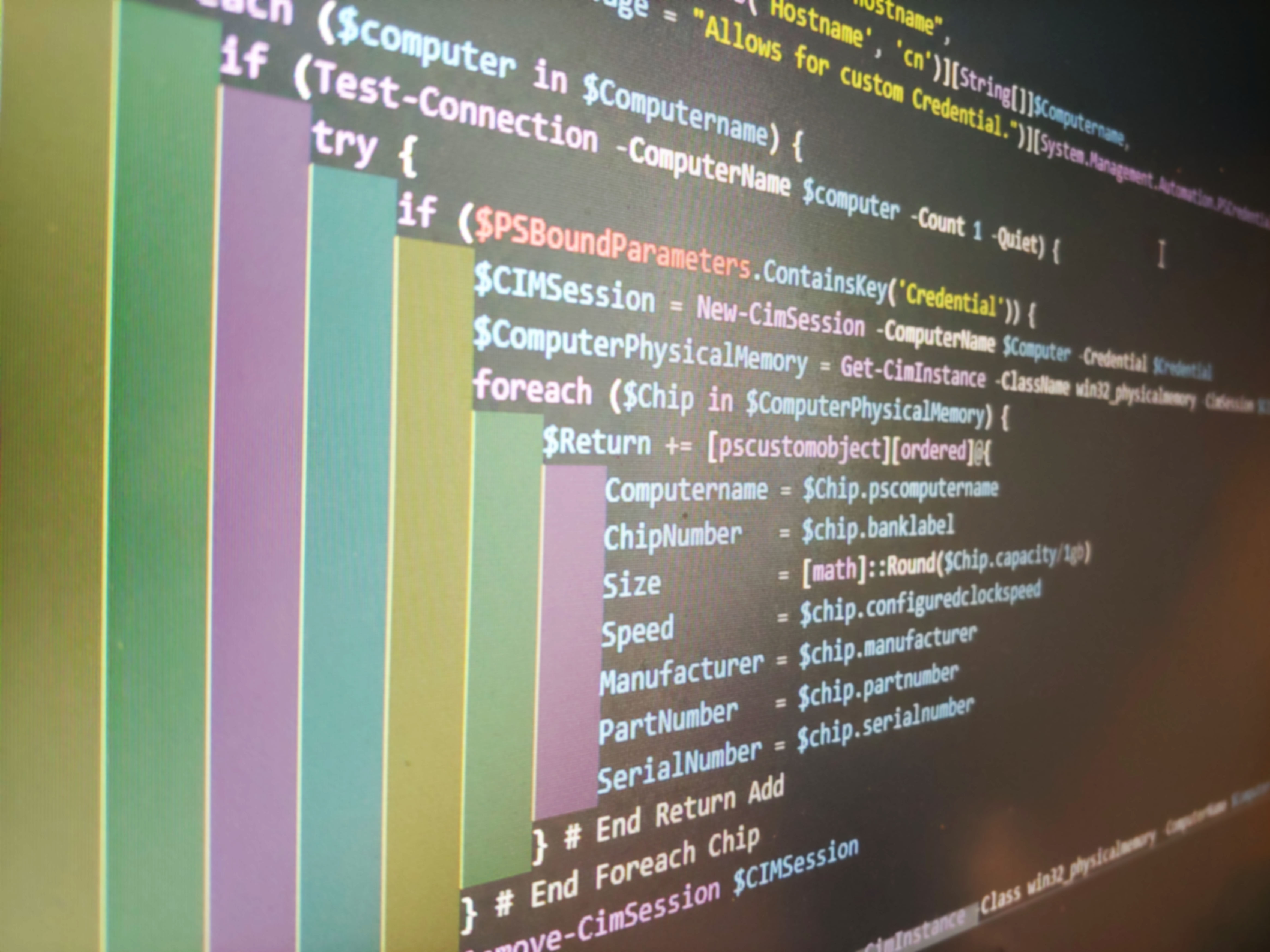
SHD – Set Mac Structure
I hate it when you get a mac address that’s not in the right format. Last week I got the mac address XX-XX-XX-XX when I needed to input it as XX:XX:XX:XX. So aggravating. So, here comes PowerShell to help. First, let’s validate the mac address. Second, let’s change out the pattern, and finally, we display the information.
Validate Mac Address
To validate a mac address we need to use a regex. I like the input to validate the pattern for us.
[parameter(Mandatory = $True)][ValidatePattern("^([0-9A-Fa-f]{2}[: \.-]){5}([0-9A-Fa-f]{2})$")][string]$MacAddress,
Let’s take a look at the validate pattern. ^([0-9A-Fa-f]{2}[: .-]){5}([0-9A-Fa-f]{2})$ The [0-9A-Fa-f] searches or the number 0-9, all the letters A,B,C,D,E,F, and the letters a,b,c,d,e,f. {2} states to look for only two of these characters. [: .-] is the next few characters we are searching for. This is the separator area of the mac address. xx:xx. From here we make sure all of this is repeated 5 times. Thus we need to make sure it’s grouped together using the preferences. ([0-9A-Fa-f){2}[: .-]). Now, this is grouped together, we want it to repeat 5 times. We don’t want it to repeat every time because the last subset does not have a separator. We do this using {5} after our group. Finally, we ask for the same thing again. ([0-9A-Fa-f]{2}). We finish it off with $.
Editing the Separators
Now we have our mac address validated we need to start building a way to replace the separators. First we need to setup the patter like above.
$Pattern = '[0-9A-Fa-f]'
Now this is the patter we are gong to look for. We want all the 0 – 9, A,B,C,D,E,F,a,b,c,d,e,f.
$Mac = $MacAddress -replace $Pattern, $Seperator
With this command we are taking the validated mac address, searching for the patter, and replacing the separators with the provided separator. Next, we can uppercase or lower case the mac address using $Mac.ToUpper() or $Mac.ToLower().
The Script
Below is the script that will make life easier for everyone. Notice, I gave the option to add the output to the clipboard.
function Set-SHDMacAddressStructure {
[cmdletbinding()]
param (
[parameter(Mandatory = $True)][ValidatePattern("^([0-9A-Fa-f]{2}[: \.-]){5}([0-9A-Fa-f]{2})$")][string]$MacAddress,
[parameter(Mandatory = $true)][String]$Seperator,
[Parameter(HelpMessage = "Changes the case")][Validateset("UpperCase", "LowerCase")]$Case,
[parameter(helpmessage = "Added mac to clipboard")][switch]$ToClipboard
)
$Pattern = '[^a-zA-Z0-9]'
$Mac = $MacAddress -replace $Pattern, $Seperator
if ($case -eq "UpperCase") {
if ($ToClipboard) {
$Mac.ToUpper() | clip
$Mac.ToUpper()
} else {
$Mac.ToUpper()
}
}
elseif ($case -eq "LowerCase") {
if ($ToClipboard) {
$Mac.ToLower() | clip
$Mac.ToLower()
} else {
$Mac.ToLower()
}
}
else {
if ($ToClipboard) {
$Mac | clip
$Mac
} else {
$Mac
}
}
}