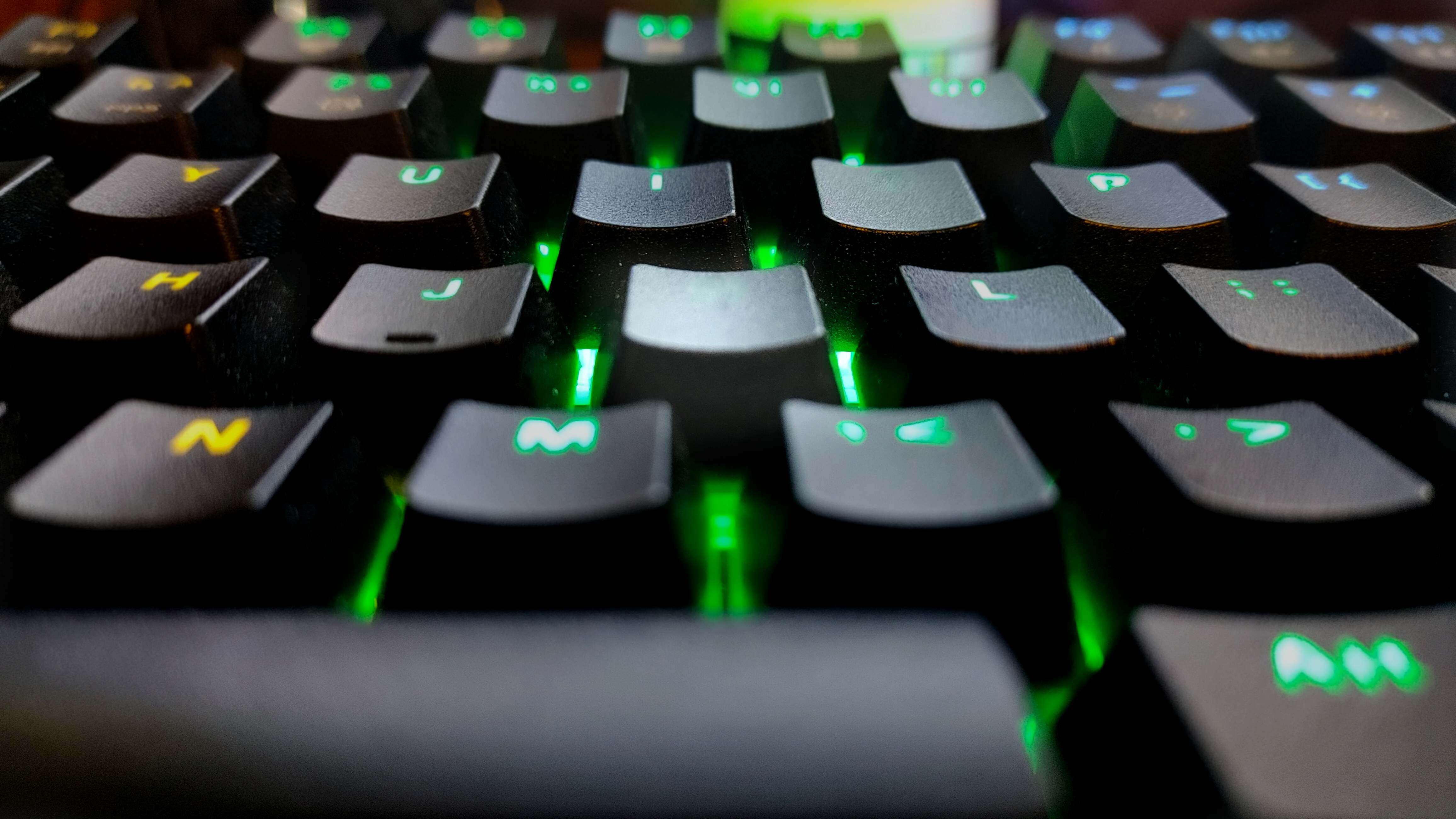
Citrix Workspace Installer Script
I don’t like working with Citrix receiver. They drive me crazy. One version doesn’t work with the other and so on and so forth. Then finding the one you need is a pain. Thankfully, the workspace is a little better at this process. Many of my clients have recently updated their back end so the new workspace will work for them. It only took a while. So, I built a script that automatically downloads the newest version and installs it accordingly. It wasn’t until later did I realize someone else did this already. But the one I made is a little better as it doesn’t run into the conflict of pulling the version number, at least in my humble opinion. This time we will start off with the script for us lazy admins. If you want to learn how it works, keep reading on.
The Script
IF (!(Test-Path c:\temp)){New-Item -Path c:\ -Name Temp -ItemType "directory"}
IF (!(Test-Path c:\temp\Citrix)) {New-Item -Path c:\temp -Name Citrix -ItemType "directory"}
$StartTime = (Get-Date).tostring("yyyy-MM-dd_hh-mm-ss")
$Logname = "C:\temp\Citrix\Install_$StartTime.log"
$DownloadFullPath = "C:\temp\Citrix\Installer_$StartTime.exe"
"Log: $($startTime): Started" > $Logname
try {
$CitrixPage = Invoke-WebRequest -UseBasicParsing -Uri ("https://www.citrix.com/downloads/workspace-app/windows/workspace-app-for-windows-latest.html") -SessionVariable websession
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Accessed" >> $Logname
} catch {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Failed to access" >> $Logname
Write-Error "Site Error: Site not accessible"
Break
}
$DownloadLink = $CitrixPage.Links | Where-Object {$_.rel -like "*CitrixWorkspaceApp.exe*"}
$URL = "Https:$($DownloadLink.rel)"
try {
Invoke-WebRequest -Uri $URL -OutFile $DownloadFullPath
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Download $URL to $DownloadFullPath" >> $Logname
} catch {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Failed to download $URL to $DownloadFullPath" >> $Logname
Write-Error "Site Error: Download Failure"
Break
}
try {
$Install = Start-Process -FilePath $DownloadFullPath -ArgumentList '/silent /forceinstall /AutoUpdateCheck=disabled /noreboot' -PassThru -ErrorAction Stop
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Install: $($LogTime): Installing $DownloadFullPath" >> $Logname
} catch {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Install: $($LogTime): $DownloadFullPath Failed to Install" >> $Logname
Write-Error "Install Error"
Break
}
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Sleep: Sleep for 420 Seconds for install" >> $Logname
Start-Sleep -Seconds 420
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Sleep: Stop Sleep" >> $Logname
$Programs = Get-CimInstance -ClassName win32_product
$Citrix = $Programs | where-object {$_.name -like "Citrix*Workspace*Browser"}
if ($null -ne $Citrix) {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Check: $($LogTime): $($Citrix.Caption) - $($Citrix.Version) Installed on: $($Citrix.Installdate.tostring())" >> $Logname
} else {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Check: $($LogTime): Install Failed" >> $Logname
}
Remove-Item -Path $DownloadFullPath -Force
The Break Down
Lets break this guy down. The first part is we are testing if the c:\temp folder exists. If it doesn’t then we will create it. Then we test if the Citrix folder exists, if it doesn’t, once again, we create it. We do this with the Test-Path for testing and the New-Item cmdlets.
IF (!(Test-Path c:\temp)){New-Item -Path c:\ -Name Temp -ItemType "directory"}
IF (!(Test-Path c:\temp\Citrix)) {New-Item -Path c:\temp -Name Citrix -ItemType "directory"}
Now we have the folders we will be using created, we need to move to creating the first log entry. We want this log to have a timestamp on it that matches the downloaded installer. To do this, we need to get the datetime first. While doing that we will create the filename of the log and the file name of the download path. This way it’s easier to work with later on in the script. We do this by using the Get-Date cmdlet. Normally the Get-Date cmdlet outputs is an object. Which isn’t very useful in a file name since it contains forbidden characters. (Not forbidden like slifer the sky dragon). A translation is required. We do this with the .tostring() method. Notice the way we format it.
- y = year
- M = Month
- d = day
- h = hour
- m = minute
- s = seconds
$StartTime = (Get-Date).tostring("yyyy-MM-dd_hh-mm-ss")
We then use the $StartTime variable inside the log name and the download pathname. This is done by a string with the variable inside of it. Next will be to create the log. We do this with a simple > which means out and create. >> means out and append. Notice in the example below we $($StartTime) we do this because the next character is a :. Inside PowerShell, you can do things like $Global:Var which tells the shell to keep that var in memory for other functions to use. This means the : is a command character. This is why we wrap the start time variable inside a $(). Powershell will only print what is inside the $(). Finally, take note of the > $Logname. We will be using $Logname more inside this script. This is why we created the variable.
$Logname = "C:\temp\Citrix\Install_$StartTime.log"
$DownloadFullPath = "C:\temp\Citrix\Installer_$StartTime.exe"
"Log: $($startTime): Started" > $Logname
Now we have the start of the log. It’s time to get the installer. In the past, we would just go to the download link and add that to a download script. However, recently Citrix changed how they download. They have tacked on an additional piece of code. Everything past the GDA is that special code they have tacked on to stop direct downloading. However, we have PowerShell on our side.
https://downloads.citrix.com/19176/CitrixWorkspaceApp.exe?__gda__=1615916903_06373f7510a0edd3a06ef41c13dbe8a7
The first thing we want to do is setup a try catch. This way we can catch errors and log them. Also we can break the script with an error message that is useful. This way if you are deploying out with something like continuum or PDQ your error message makes sense. Inside the try, we want to get the webpage itself. Then log that we grabbed information. The cmdlet to get the website is Invoke-webrequest. In the below example I am using the -usebasicparsing because it’s more compatible with websites and with systems. My goal is to launch this thing to 100+ machines. The -Uri is for the website itself and finally, we use the -sessionvariable as a websession. This allows us to grab data easier, especially if it’s auto-generated, like in this case.
$CitrixPage = Invoke-WebRequest -UseBasicParsing -Uri ("https://www.citrix.com/downloads/workspace-app/windows/workspace-app-for-windows-latest.html") -SessionVariable websession
After we grab the website, we have to log the event. We do the same thing we did with $StartTime and place it in the file we created a few moments ago.
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Accessed" >> $Logname
If these commands fail for whatever reason, the website is down, the internet is blocking, anything, we need to know that the site can’t be reached. This is why we have a log. We create the same as the $logime but this time we also add a write-error and a break command. The write-error command will send an error to a deployment software, This way we know what’s going on. The break command breaks the script at that point and doesn’t continue.
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Failed to access" >> $Logname
Write-Error "Site Error: Site not accessible"
Break
Lets put them together inside the try catch so you can see what it looks like.
try {
$CitrixPage = Invoke-WebRequest -UseBasicParsing -Uri ("https://www.citrix.com/downloads/workspace-app/windows/workspace-app-for-windows-latest.html") -SessionVariable websession -DisableKeepAlive
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Accessed" >> $Logname
} catch {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Failed to access" >> $Logname
Write-Error "Site Error: Site not accessible"
Break
}
Now we have the website itself inside a variable. It’s time to find what we need. The $CitrixPage contains different elements inside of it. Each item can give you information. The Rawcontent is just like it sounds, raw content of the page. While status code can give you information about if the site is up or what condition it is in. In this case, we will be looking at the links and status code. We check if the site has a good status of 200, if it doesn’t, then we don’t want to battle that battle. Thus we log and break like before. If it does, however, we want to take apart the links and find the one that contains the exe that we need. We do this with a where-object cmdlet. We search the .rel for the *CitrixWorkspaceApp.exe. Because the .links sometimes produces incomplete links, we have to build them. That’s the second step is to build the link. We will wrap the outcome inside an https: string.
if ($CitrixPage.statuscode -eq 200) {
$DownloadLink = $CitrixPage.Links | Where-Object {$_.rel -like "*CitrixWorkspaceApp.exe*"}
$URL = "Https:$($DownloadLink.rel)"
} else {
"Site: $($LogTime): Site Status Code $($CitrixPage.StatusCode)" >> $Logname
Write-Error "Site Error: Status Code $($CitrixPage.StatusCode)"
Break
}
Now we have the custom URL for the download, we need to download the file itself. Remember the $DownloadFullPath we created a while ago. It’s time to use it. We will be using the invoke-webrequest once again as well. This time we will use the -OutFile cmdlet. This cmdlet of invoke-webrequest will download the file as requested from the url provided. Of course, we want to wrap all of this inside of a try catch. This way we can log correctly and break as needed.
try {
Invoke-WebRequest -Uri $URL -OutFile $DownloadFullPath
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Download $URL to $DownloadFullPath" >> $Logname
} catch {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Site: $($LogTime): Failed to download $URL to $DownloadFullPath" >> $Logname
Write-Error "Site Error: Download Failure"
Break
}
Now we have the installer to work with. The filename has the same time/date stamp as the log file so we can compare if the script doesn’t finish up correctly. Next we will start the process of another try catch to install the program. The command we will use is the Start-Process command. We start the $DownloadFullPath for the file name. We want this thing to be quiet and overwrite everything else there. Citrix, if given the /forceinstall will force the install by uninstalling the last version. Finally we tell it not to reboot with the /noreboot. Once we get past the arguments, we want to make sure we have the information from this thus we put the -passthru flag. This will allow us to store the information into a variable if we want to use that information later. The final part of the command is the -erroraction. We want this thing to stop if it hits an error. This way we know that something is broken. Then we log accordingly and catch accordingly like above.
try {
$Install = Start-Process -FilePath $DownloadFullPath -ArgumentList '/silent /forceinstall /AutoUpdateCheck=disabled /noreboot' -PassThru -ErrorAction Stop
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Install: $($LogTime): Installing $DownloadFullPath" >> $Logname
} catch {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Install: $($LogTime): $DownloadFullPath Failed to Install" >> $Logname
Write-Error "Install Error"
Break
}
We are almost done! This program takes an average of 5 minutes on older machines to install. Thus we sleep for 7 minutes. To do this we use the command Start-Sleep and set the -seconds to 420 seconds. We also make sure we log this information.
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Sleep: Sleep for 420 Seconds for install" >> $Logname
Start-Sleep -Seconds 420
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Sleep: Stop Sleep" >> $Logname
As we are not in a hurry, we use the PowerShell command Get-CimInstance to get the products and sort through that to find the Citrix Workplace Browser using the where-object cmdlet.
$Programs = Get-CimInstance -ClassName win32_product
$Citrix = $Programs | where-object {$_.name -like "Citrix*Workspace*Browser"}
Finally we check to see if the install was successful or not. This is done with a simple $null -ne $something. We do it this way because we first load nothing and start to compare nothing to something. if something is there, then we know the statement is true and stop processing. Very simple concept. If $Citrix does contain something we log that the install was successful and remove the installer. If we find $Citrix is $null, then we log the error and error out once again.
if ($null -ne $Citrix) {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Check: $($LogTime): $($Citrix.Caption) - $($Citrix.Version) Installed on: $($Citrix.Installdate.tostring())" >> $Logname
Remove-Item -Path $DownloadFullPath -Force
} else {
$LogTime = (Get-date).tostring("yyyy:MM:dd-hh:mm:ss")
"Check: $($LogTime): Install Failed" >> $Logname
Write-Error "Install: Install not complete."
break
}
Improvements
With all good scripts, there is always room for improvement. The one that is blaring is the waiting for the install. This should really be a loop checking files or a registry key. If the file or key is not present, then continue to way 30 seconds. This would speed up the process as some computers process faster while others do not.
The second is the get-ciminstance because this is a slow command. We can improve the speed of this command by targeting once again either a file or a registry key instead. This way we can prove it was installed without the 30 to 60 second wait for the get-ciminstance to do its thing.
As always, If you have any questions, feel free to ask.