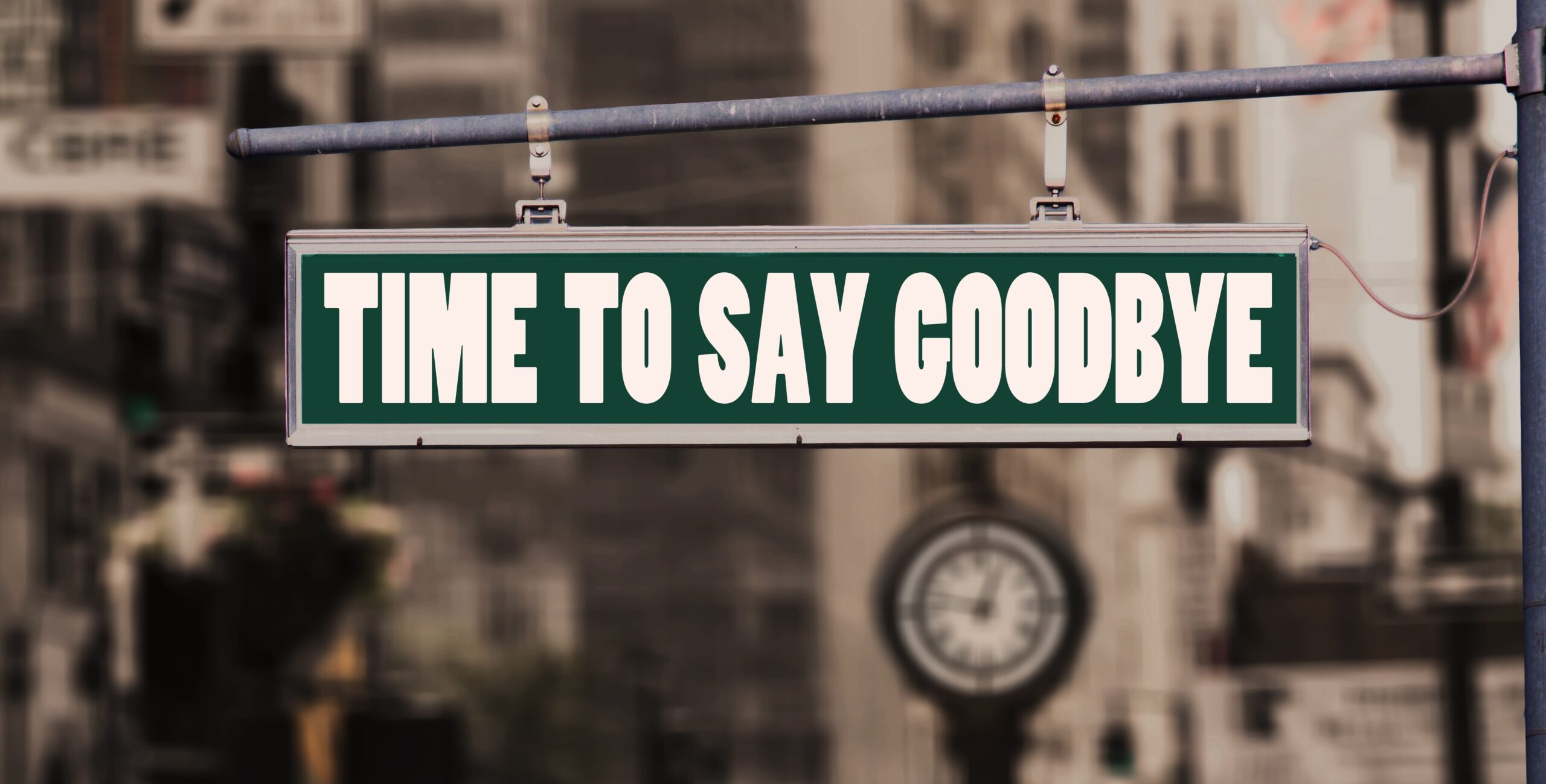
SHD – Disable User
My last blog was about how to find the disabled user OU. Now we will go over how I disable users and move them around to the disabled OU. The next blog will combine all this together and remove inactive accounts that are within a set OU. Let’s rock this!
First first step is to get the disabled OU. In this script, you can either add it yourself or allow it to find the OU for you. It uses the Find-SHDDisabledUsersOU to find it for you.
The next step is to get the user info. We do this with the simple command, Get-ADuser.
$TargetUser = Get-ADUser -Identity $user -Properties *
Now, I believe in removing a user from all Groups that it can be removed from. By default, the default group can’t be removed only reassigned. So, I will not go through the process of reassigning the default group. If that is part of your disabling process, you may modify this code to add it. So, lets remove all the groups.
$Targetuser.memberof | foreach-object { Remove-ADGroupMember -Identity $_.DistinguishedName -Members $targetuser.samaccountname -Confirm:$false }
We list all the memberof of the target user. Then we start a foreach-object. Inside the foreach-object we have a remove-adgroupmember. Notice the Identity tag is using the $_.DistinguishedName. This prevents errors and makes things generally faster. Only by a few milliseconds. Those milliseconds do add up over time. Then we tell it to remove the Samaccountname of the target user. The final step is what devides automation from “Damn confirmation boxes” -Confirm:$False. This flag surpresses the need to confirm everything. Thus clearing out all the groups. On average this takes about 1 second for me whie on network.
The next step is to clear all the information from the user account. 90% of the time the users that do come back, come back as something else. Most of the time, they don’t come back. We do this with a Set-ADuser command.
Set-ADUser -Identity $TargetUser.samaccountname -Department '' -Title '' -City '' -Company '' -Country '' -Description '' -Division '' -EmailAddress '' -EmployeeID '' -EmployeeNumber '' -Fax '' -Enabled $false -HomeDirectory '' -HomeDrive '' -HomePage '' -HomePhone '' -OtherName '' -Manager '' -LogonWorkstations '' -MobilePhone '' -Office '' -OfficePhone '' -Organization '' -POBox '' -PostalCode '' -ProfilePath '' -ScriptPath '' -State '' -StreetAddress ''
The set-aduser command is straight forward. It uses the Target user samaccountname and clears anything I can. Now we will reset the password.
$Password = -join ((32..95) + (97..126) | Get-Random -Count 90 | ForEach-Object { [char]$_ })
Set-ADAccountPassword -Identity $TargetUser.Samaccountname -Reset -NewPassword (ConvertTo-SecureString -AsPlainText -String $Password -Force)
We want the length of the password to be 90 characters long. We then start a loop and join the characters we want (Password-safe characters) and get the random of these characters. Then We loop through that to get our password. After that, we push this password into the password reset command. Set-ADAccountPassword.
Notice in the password reset command, we use the flag Reset. Then NewPassword. Notice with the newpassword tag we have a converto-securestring.
ConvertTo-SecureString -AsPlainText -String $Password -Force
We run the text as plain text and force the string. This gives us a secure password to use. As the admin, we don’t need to know the password as we can reset it later.
Now we disable the account with disable-adaccount.
Disable-ADAccount -Identity $TargetUser.samaccountname
Disable-ADAccount is very straight forward. You give it the identity of the user with the targetuser.samaccountname and your done.
The final step is to move the user to the Disabled OU. We do this with Move-ADObject.
Move-ADObject -Identity $TargetUser.samaccountname -TargetPath $DisabledOU
We are telling the system to move the AD user to the disabled ou path. Now we are done. From here you can have an email sent or any other notification information if you want to see the output. Ok, it’s time. lets put it all together.
The Script
Function Disable-SHDUser {
<#
.SYNOPSIS
.DESCRIPTION
.PARAMETER
.EXAMPLE
.INPUTS
.OUTPUTS
.NOTES
.LINK
#>
[cmdletbinding()]
param (
[Parameter(
ValueFromPipeline = $True,
ValueFromPipelineByPropertyName = $True,
HelpMessage = "Provide the target hostname",
Mandatory = $true)][Alias('Hostname', 'cn')][String[]]$Username,
[parameter(HelpMessage = "Moves to this OU if provided, If not, finds disable ou and moves it.")][string]$OU,
[Parameter(HelpMessage = "Allows for custom Credential.")][System.Management.Automation.PSCredential]$Credential
)
if ($PSBoundParameters.ContainsKey('Credential')) {
if ($PSBoundParameters.ContainsKey('OU')) {
$DisabledOU = $OU
} else {
$DisabledOU = Find-SHDDisabledUsersOU -Credential $Credential
}
foreach ($user in $Username) {
$TargetUser = Get-ADUser -Identity $user -Properties * -Credential $Credential
$Targetuser.memberof | foreach-object { Remove-ADGroupMember -Credential $Credential -Identity $_.DistinguishedName -Members $targetuser.samaccountname -Confirm:$false }
Set-ADUser -Identity $TargetUser.samaccountname -Department '' -Title '' -City '' -Company '' -Country '' -Description '' -Division '' -EmailAddress '' -EmployeeID '' -EmployeeNumber '' -Fax '' -Enabled $false -HomeDirectory '' -HomeDrive '' -HomePage '' -HomePhone '' -OtherName '' -Manager '' -LogonWorkstations '' -MobilePhone '' -Office '' -OfficePhone '' -Organization '' -POBox '' -PostalCode '' -ProfilePath '' -ScriptPath '' -State '' -StreetAddress '' -Credential $Credential
$Password = -join ((32..95) + (97..126) | Get-Random -Count 90 | ForEach-Object { [char]$_ })
Set-ADAccountPassword -Identity $TargetUser.Samaccountname -Reset -NewPassword (ConvertTo-SecureString -AsPlainText -String $Password -Force) -Credential $Credential
Disable-ADAccount -Identity $TargetUser.samaccountname -Credential $Credential
Move-ADObject -Identity $TargetUser.samaccountname -TargetPath $DisabledOU -Credential $Credential
}
}
else {
if ($PSBoundParameters.ContainsKey('OU')) {
$DisabledOU = $OU
} else {
$DisabledOU = Find-SHDDisabledUsersOU
}
foreach ($user in $Username) {
$TargetUser = Get-ADUser -Identity $user -Properties *
$Targetuser.memberof | foreach-object { Remove-ADGroupMember -Identity $_.DistinguishedName -Members $targetuser.samaccountname -Confirm:$false }
Set-ADUser -Identity $TargetUser.samaccountname -Department '' -Title '' -City '' -Company '' -Country '' -Description '' -Division '' -EmailAddress '' -EmployeeID '' -EmployeeNumber '' -Fax '' -Enabled $false -HomeDirectory '' -HomeDrive '' -HomePage '' -HomePhone '' -OtherName '' -Manager '' -LogonWorkstations '' -MobilePhone '' -Office '' -OfficePhone '' -Organization '' -POBox '' -PostalCode '' -ProfilePath '' -ScriptPath '' -State '' -StreetAddress '' -Credential $Credential
$Password = -join ((32..95) + (97..126) | Get-Random -Count 90 | ForEach-Object { [char]$_ })
Set-ADAccountPassword -Identity $TargetUser.Samaccountname -Reset -NewPassword (ConvertTo-SecureString -AsPlainText -String $Password -Force)
Disable-ADAccount -Identity $TargetUser.samaccountname
Move-ADObject -Identity $TargetUser.samaccountname -TargetPath $DisabledOU
}
}
}