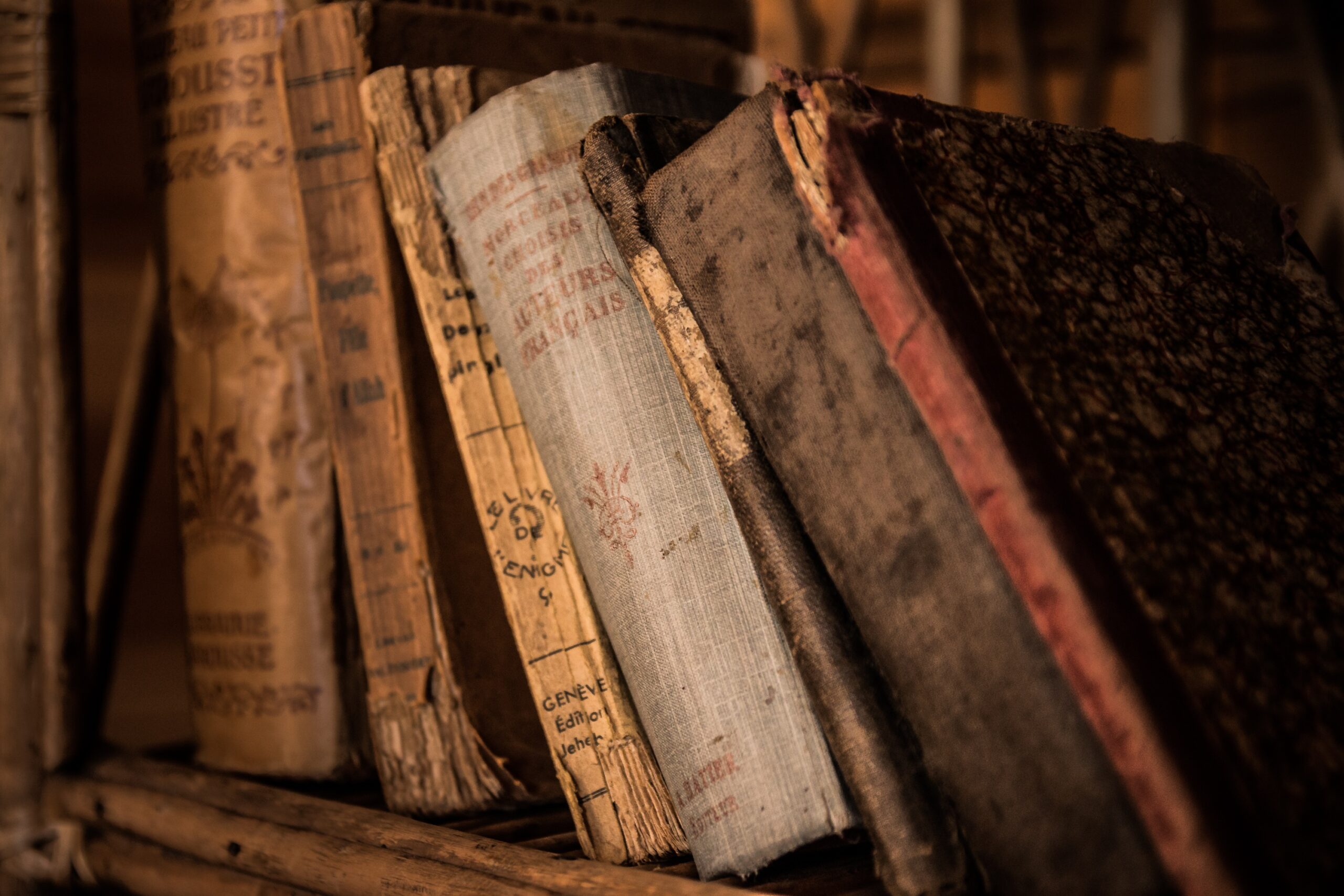
SHD – Quickbook Search
This past week I needed to find all of the quickbook files on a computer without accessing quickbooks itself. The core of the script is a simple Get-Childitem command looped for each logical disk on the machine. Looking for one of the four main extensions for quickbooks. The four extensions are as follows
Name | Extension |
Company Files | qbw |
Backup Files | qbb |
Portable Files | qbm |
Bank Statement files | qbo |
The Script
function Search-QuickbookFiles {
[cmdletbinding()]
Param (
[parameter(Mandatory = $True)][Validateset("Company Files", "Backup Files", "Portable Files", "Bank Statement Files")][string]$FileType
)
if ($FileType -like "Company Files") { $EXT = "QBW" }
if ($FileType -like "Backup Files") { $EXT = "QBB" }
if ($FileType -like "Portable Files") { $EXT = "QBM" }
if ($FileType -like "Bank Statement Files") { $EXT = "QBO" }
$Disks = Get-CimInstance -ClassName win32_logicaldisk
$QB = foreach ($Disk in $Disks) {
Get-ChildItem -Path "$($Disk.DeviceID)\" -Filter "*.$EXT" -Recurse | Select-Object FullName,Length,LastWriteTime
}
$QB
}
$DateTime = (Get-Date).tostring("yyyy-MM-dd_hh-mm-ss")
if (!(Test-Path C:\temp)) {mkdir c:\temp}
Search-QuickbookFiles -FileType 'Company Files' | Export-Csv "c:\temp\$($Env:COMPUTERNAME)_CompanyFiles_$($DateTime).CSV"
Search-QuickbookFiles -FileType 'Backup Files' | Export-Csv "c:\temp\$($Env:COMPUTERNAME)_BackupFiles_$($DateTime).CSV"
Search-QuickbookFiles -FileType 'Portable Files' | Export-Csv "c:\temp\$($Env:COMPUTERNAME)_PortableFiles_$($DateTime).CSV"
Search-QuickbookFiles -FileType 'Bank Statement Files' | Export-Csv "c:\temp\$($Env:COMPUTERNAME)_BankStatementFiles_$($DateTime).CSV"
The Breakdown
Since I want this to be a little easier to use, I broke it down between the file types with a validate set parameter. This way you can choose which extension you want. Then I go through each extension and make an if statement for each one matching up the extension.
Next we get the disks using the Get-CimInstance -classname win32_logicaldisk. This grabs all the mapped drives, local drives, and anything else that has a drive letter.
Now we loop through those disks and search the root of each drive for any files with the extension we choose. We select the fullname as this gives us the full path. I also like having file size and last write time to determine if the file is valid still. Once we go through this loop we display the information.
Improvements
I can add remote computers to this setup.
That’s it, if you have any questions feel free too reach out.