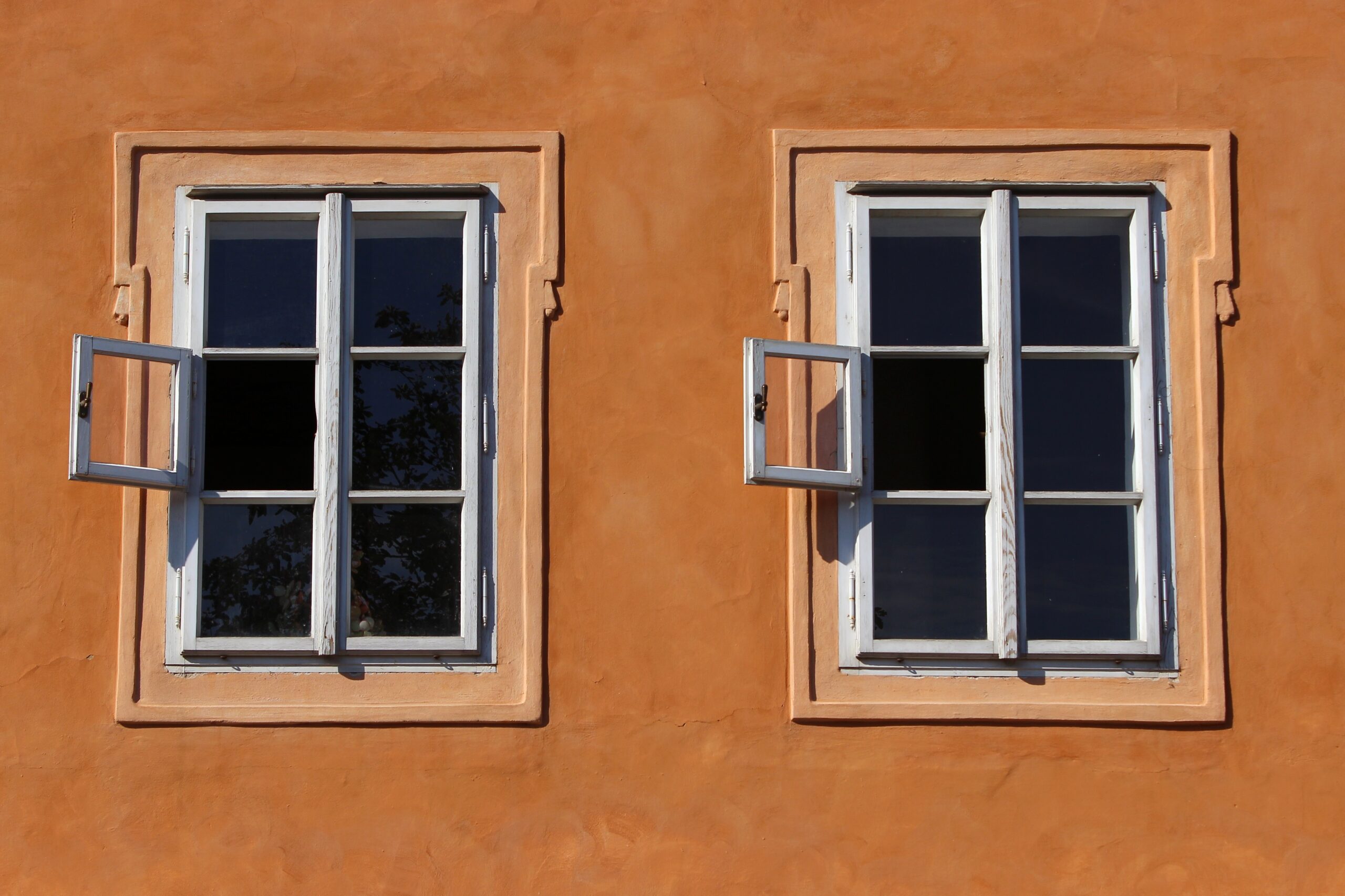
Copy A User’s Group to Other Users
Often times I am asked to give user a the same rights as user b. Since everything is setup with groups, this is easy to do. All I have to do is copy all the security groups to the new users. Normally I would use the command Get-ADPrincipalGroupMembership whoever, that has become less trust worthy over time for us. So I made a different approach.
$Groups = (Get-ADUser -Identity $SourceUser -Properties memberof).memberof -Replace '^cn=([^,]+).+$', '$1' | Sort-Object | ForEach-Object { (Get-ADGroup -Filter "name -like '$_'")}
The above command grabs the source users member of and filters it out for just the name. Then it loops through each and gets the group name. From here we can filter even farther to get the security and then loop those and added the single member making a one liner, but I want this thing to be bigger and better. A good function that can do distribution, security, universal, global and domain local groups to more than one single user with credentials or not. I want this thing to be nice. Here is the heart beat of the command:
foreach ($Target in $TargetUser) {
$Groups | ForEach-Object {
try {
if ($PSBoundParameters.ContainsKey('Credential')) {
Write-Verbose "Add $($_.Samaccountname) a $($_.GroupCategory.tostring()) a $($_.GroupScope.tostring()) to $Target"
Add-ADGroupMember -Identity $_.samaccountname -Members $Target -Credential $Credential
} else {
Write-Verbose "Add $($_.Samaccountname) a $($_.GroupCategory.tostring()) a $($_.GroupScope.tostring()) to $Target"
Add-ADGroupMember -Identity $_.samaccountname -Members $Target
}
} catch {
Write-Verbose "Failed to add $($_.Samaccountname) to $Target"
Write-Warning "$_ could not apply to $Target"
}
}
}
We target each user with this information. Then we add the group accordingly. So, how do you split the Security group vs Distribution group? We do this by using PSboundparameters and a Where-object group. So we declare a parameter in the parameter area and then ask if it is being used. If it is, we use the where object to search for the groupcategory.
$Groups = (Get-ADUser -Identity $SourceUser -Properties memberof).memberof -Replace '^cn=([^,]+).+$', '$1' | Sort-Object | ForEach-Object { (Get-ADGroup -Filter "name -like '$_'")}
if ($PSBoundParameters.ContainsKey('GroupCategory')) {$Groups = $Groups | Where-Object {$_.GroupCategory -like "$GroupCategory"}}
if ($PSBoundParameters.ContainsKey('GroupScope')) {$Groups = $Groups | Where-Object {$_.GroupScope -like "$GroupScope"}}
Lets put it all together shall we, so you can get back on with your day.
Function Copy-SHDUserGroupToUser {
[cmdletbinding()]
param (
[Parameter(
ValueFromPipeline = $True,
ValueFromPipelineByPropertyName = $True,
HelpMessage = "Enter a users Name",
Mandatory = $true)][String]$SourceUser,
[Parameter(HelpMessage = "Target User", Mandatory = $True)][string[]]$TargetUser,
[parameter(Helpmessage = "Group Category")][validateset("Security","Distribution")]$GroupCategory,
[parameter(Helpmessage = "Group Scope")][validateset("Universal","Global","DomainLocal")]$GroupScope,
[Parameter(HelpMessage = "Allows for custom Credential.")][System.Management.Automation.PSCredential]$Credential
)
$Groups = (Get-ADUser -Identity $SourceUser -Properties memberof).memberof -Replace '^cn=([^,]+).+$', '$1' | Sort-Object | ForEach-Object { (Get-ADGroup -Filter "name -like '$_'")}
if ($PSBoundParameters.ContainsKey('GroupCategory')) {$Groups = $Groups | Where-Object {$_.GroupCategory -like "$GroupCategory"}}
if ($PSBoundParameters.ContainsKey('GroupScope')) {$Groups = $Groups | Where-Object {$_.GroupScope -like "$GroupScope"}}
foreach ($Target in $TargetUser) {
$Groups | ForEach-Object {
try {
if ($PSBoundParameters.ContainsKey('Credential')) {
Write-Verbose "Add $($_.Samaccountname) a $($_.GroupCategory.tostring()) a $($_.GroupScope.tostring()) to $Target"
Add-ADGroupMember -Identity $_.samaccountname -Members $Target -Credential $Credential
} else {
Write-Verbose "Add $($_.Samaccountname) a $($_.GroupCategory.tostring()) a $($_.GroupScope.tostring()) to $Target"
Add-ADGroupMember -Identity $_.samaccountname -Members $Target
}
} catch {
Write-Verbose "Failed to add $($_.Samaccountname) to $Target"
Write-Warning "$_ could not apply to $Target"
}
}
}
}
Examples
Copy-SHDUserGroupToUser -SourceUser bobtheuser -TargetUser test1,test2,test3 -GroupCategory Security -GroupScope Global -Verbose
This will add all of bobtheuser’s global security groups to test1, test2, and test3 users and verbose the info out.
Copy-SHDUserGroupToUser -SourceUser bobtheuser -TargetUser test1,test2,test3
This will add all the bobtheuser’s groups to test1, test2, and test3.