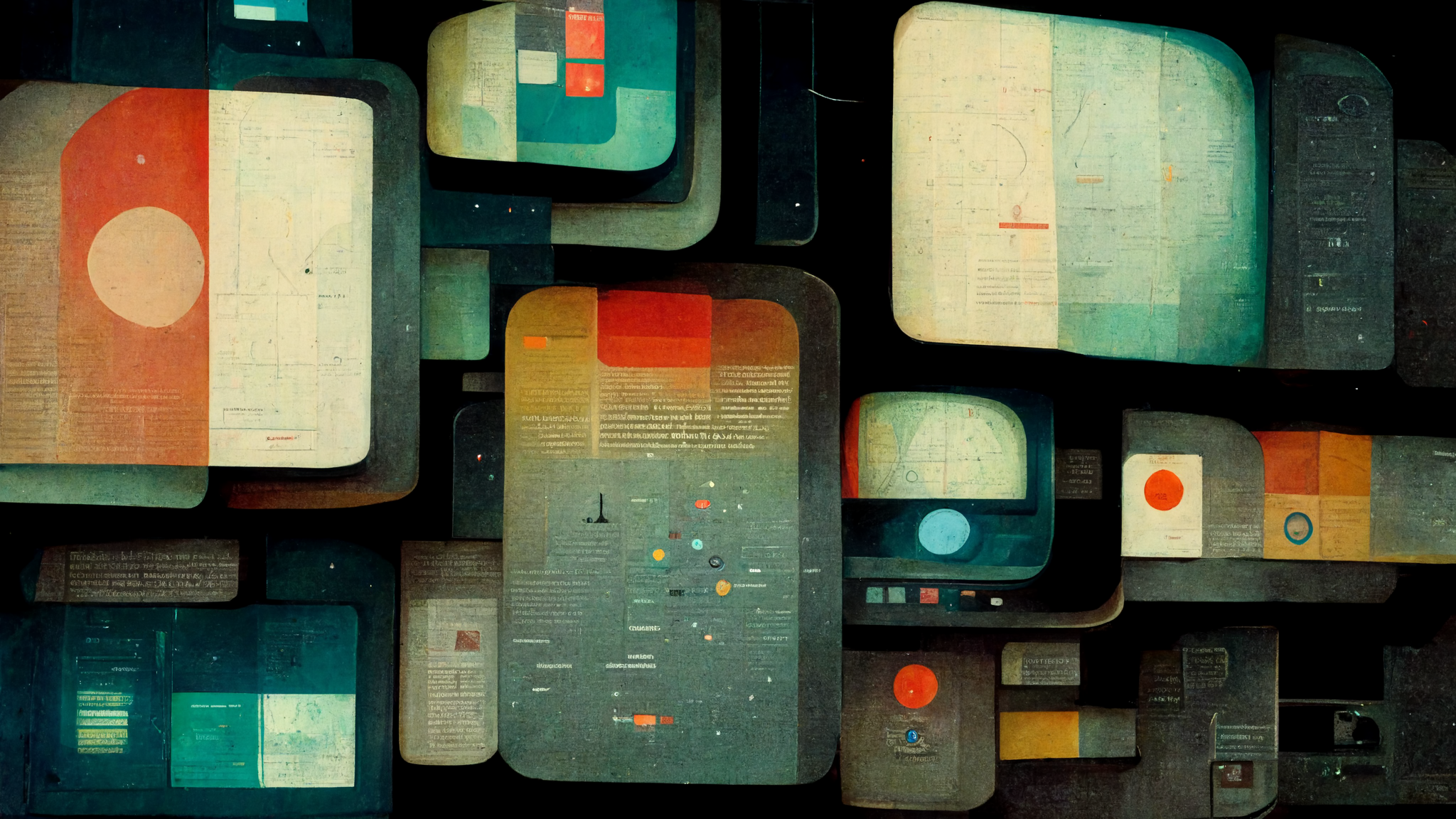
Get Client Information From your Unifi Controller
Today I would like to go over how to get client information from your Unifi Controller. We will first connect to our Unifi Controller with Powershell using the Unifi API. Then from there we will pull each site and create a list of different sites. Finally, we will use the site information to pull the client information from the controller.
Powershell and API the Unifi Controller
The first step is to create a connection with our PowerShell. We will need a few items:
- User Account that Has at least read access
- The IP address of our controller
- The API port.
- An Unifi Controller that is configured correctly. (Most are now)
Ask your system administrator to create an account with read access. Check your configuration for the IP addresses and the Port numbers. By default, the API port is 8443. Now we have those pieces of information, its time to discuss what is needed.
Connecting to the API
To connect to the Unifi controller API, you need a Cookie. This is not like how we connected to the Graph API in our previous blog. To get this cookie, we will need to execute a post. The API URL is the IP address followed by the port number, API and login. Very standard.
$uri = "https://$($IPAddress):8443/api/login"
We will need to create a header that accepts jsons. To do this we simply say accept equal application/json.
$headers = @{'Accept' = 'application/json' }
Now we will create our Parameters for the body. Since this is going to be a Post command, we have to have a body. This is where we will place our Username and Password. We are going to build out a Hashtable and then convert that into a json since the API is expecting a json. We will use the convertto-json command to accomplish this step.
$params = @{
'username' = $username;
'password' = $Password;
}
$body = $params | ConvertTo-Json
Now we have our Body built out, it’s time to create a session. We will use the invoke-restmethod command to do this. We feed the URI we made a few steps back. The body will be the body we just built out. The content type will be ‘application/json’. Our headers will be the header we made a few steps back. We will skip the certificate check unless we are using a cert. Most of the time we are not. Finally, the secret sauce. We want a session. Thus, we use the Session Variable and give it a session name. Let’s go with S for the session.
$response = Invoke-RestMethod -Uri $uri `
-Body $body `
-Method Post `
-ContentType 'application/json' `
-Headers $headers `
-SkipCertificateCheck `
-SessionVariable s
Grabbing Sites
Now we have our Session cookie, its’ time to grab some information. We will use the same headers but this time we will use the Session Name, S. The URL for sites is /api/Self/Sites.
$uri = "https://$($IPaddress):8443/api/self/sites"
This URL will get the sites from your Unifi Controller. Once again we will use the Invoke-RestMethod to pull the data down. This time we are using a get method. The same Content type and headers will be used. Along with the skip certificate check. The Session Variable is going to be the Web Session and we will use the $S this time.
$sites = Invoke-RestMethod -Uri $uri `
-Method Get `
-ContentType 'application/json' `
-Headers $headers `
-SkipCertificateCheck `
-Websession $s
The Sites variable will contain two items. a meta and a data variable. Everything we will need is inside the Data variable. To access it we can use the $sites.data to see all the data.
Here is an example of what the data will look like for the sites.
_id : 5a604378614b1b0c6c3ef9a0
desc : A descriptoin
name : Ziipk5tAk
anonymous_id : 25aa6d32-a165-c1d5-73a0-ace01b433c14
role : admin
Get Client Information from your Unifi Controller
Now that we have the sites, we can go one at a time and pull all the clients from that site. We will do this using a Foreach loop. There is something I want to point out first. Remember, the data that is returned is two variables, two arrays. The first one is Meta. Meta is the metadata of the session itself. It’s something we don’t need. We do need the Data. For our foreach loop, we will pull directly from the data array. We want to push everything in this loop into a variable. This way we can parse out useful data, sometime to much data is pointless.
$Clients = Foreach ($Site in $Sites.Data) {
#Do something
}
Without using the $Sites.Data we will have to pull the data from the command itself. This can cause issues later if you want to do more complex things.
The URL we will be using is the /api/s/{site name}/stat/sta. We will be replacing the Site name with our $site.name variable and pushing all that into another Uri.
$Uri = "https://$($IPaddress):8443/api/s/$($Site.name)/stat/sta"
Then we will execute another invoke-restmethod. Same as before, we use the same headers, content type, and web session. The only difference is we wrap up the command inside preferences. This way we can pull the data directly while the command executes. Less code that way.
(Invoke-RestMethod -Uri $Uri `
-Method Get `
-ContentType 'application/json' `
-Headers $headers `
-SkipCertificateCheck `
-Websession $s).data
Each time the command runs, it will release the data that we need and that will all drop into the $Clients variable. From here, we pull the information we want. The information that the client’s produce includes, the match addresses, times, IP addresses, possible names, IDs and more. So, it’s up to you at this point to pick and choose what you want.
The Script
The final script is different because I wanted to add site names and data to each output. But here is how I built it out. I hope you enjoy it.
function Get-UnifiSitesClients {
param (
[string]$IPaddress,
[string]$username,
[string]$portNumber,
[string]$Password
)
$uri = "https://$($IPaddress):$portNumber/api/login"
$headers = @{'Accept' = 'application/json' }
$params = @{
'username' = $username;
'password' = $Password;
}
$body = $params | ConvertTo-Json
$response = Invoke-RestMethod -Uri $uri `
-Body $body `
-Method Post `
-ContentType 'application/json' `
-Headers $headers `
-SkipCertificateCheck `
-SessionVariable s
$uri = "https://$($IPaddress):$portNumber/api/self/sites"
$sites = Invoke-RestMethod -Uri $uri `
-Method Get `
-ContentType 'application/json' `
-Headers $headers `
-SkipCertificateCheck `
-Websession $s
$Return = Foreach ($Site in $sites.data) {
$Uri = "https://$($IPaddress):$portNumber/api/s/$($Site.name)/stat/sta"
$Clients = (Invoke-RestMethod -Uri $Uri `
-Method Get `
-ContentType 'application/json' `
-Headers $headers `
-SkipCertificateCheck `
-Websession $s).data
Foreach ($Client in $Clients) {
[pscustomobject][ordered]@{
Site = $Site.name
SiteDescritption = $Site.desc
OUI = $client.OUI
MacAddress = $client.mac
IPAddress = $Client.IP
SwitchMac = $client.sw_mac
SwitchPort = $client.sw_port
WireRate = $client.wired_rate_mbps
}
}
}
$return
}
Additional Resource