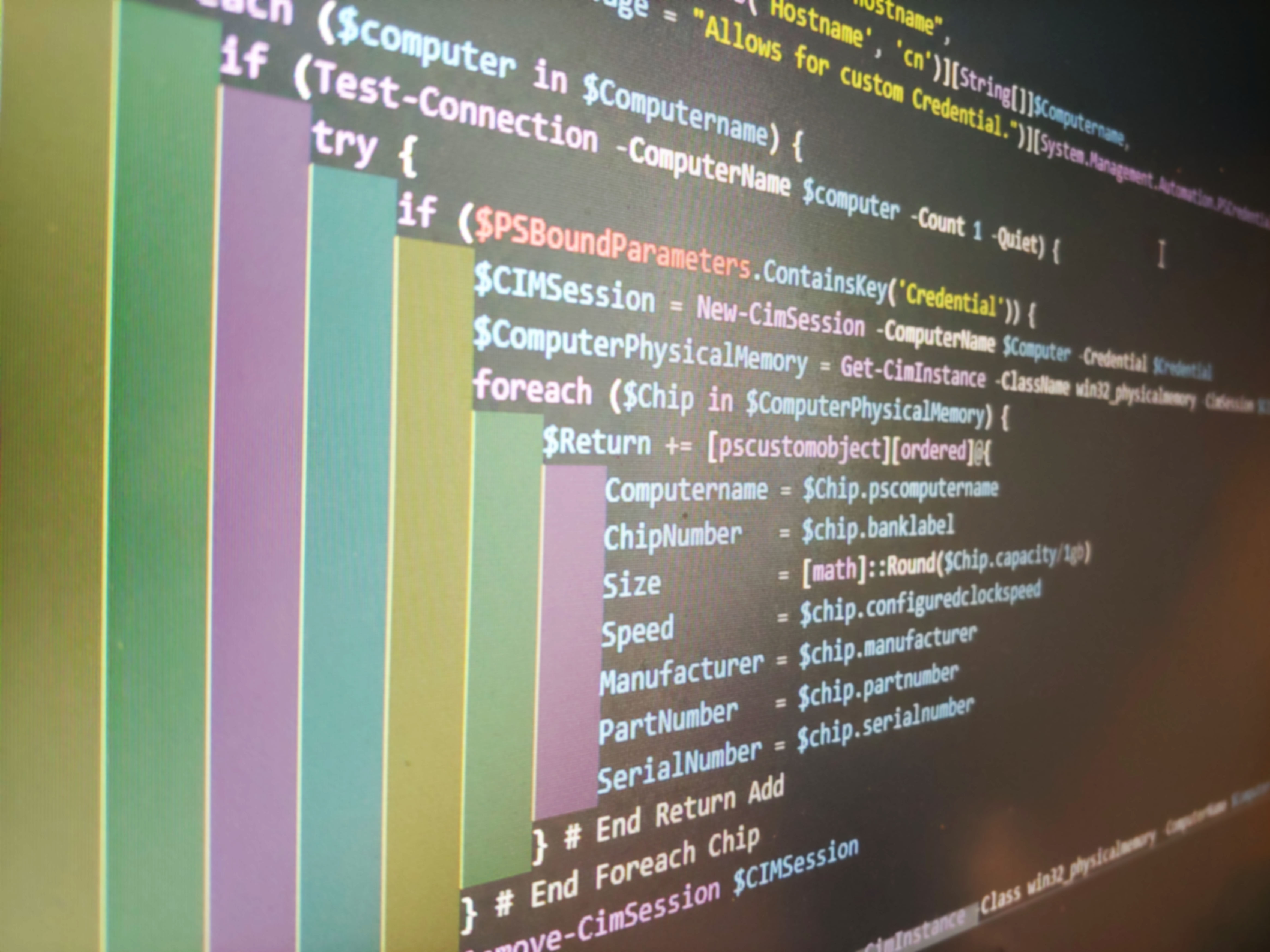
SHD Array To Line Of Text
Often times I need to put an array into a line of text. It can take a while. So I made a powershell to help me make powershells. This little guy takes a single array and turns it into a line of text that I can use in a parameter (“A”,”B”,”C”).
The Break Down
The first part of the code is the parameter block that Grabs the array. This is a simple input. We make sure the array is mandatory.
param (
[parameter(HelpMessage = "Input of Array", Mandatory = $true)][array]$TheArray
)
Next we create a blank line of text that we will be adding everything else to.
$Line = ""
I’m a little anal when it comes down to my outputs. I like everything to be sorted. Its just cleaner and easier for me to understand after the fact. So I sort the Inputs and put the input back into the inputs.
$TheArray = $TheArray | Sort-Object
Now we create the meat and potato of the script. The for loop. We will do a foreach loop in this case. Makes things easier to work with. We put each object into the $Line string with a +=.
foreach ($Info in $TheArray) {
$Line += """$Info"","
}
Lets take a look at the three ” in front of the $Info variable. “””$Info””,” Inorder to have a qoute, you must have a qoute around it inside a string. “$Info” will just put the value info into the string. But placing a ““” adds “$info as part of the string. Doing the same with the end “” does the same. Then adding the , helps build the string.
After the loop is finished, we are left with a odd , mark. The best way to handle that is to remove it. I do this by pushing the value line into it self while removing the last character with substring.
$Line = $Line.Substring(0, $Line.Length - 1)
Here the substring starts at character 0, and counts to the last character of the value line – 1. Basically, we don’t need that last one. So, everything else is pushed back into $Line.
Next we add the lazy man touch and push the string of text into the clip board to be used else where. Life is good. We do this by using the command clip
$Line | Clip
Finally, we display the information to the screen with a write-host command letting the end user know it’s inside their clipboard.
Write-Host "Input is on in your clipboard: $Line"
It’s a simple little script that has saved me on hours worth of work. I use this little guy to create a “Create PDQ deployment script” script. A powershell script that builds powershell scripts.
The Script
I would be amissed if I didn’t post the script, so here you go.
Function Convert-SHDArraytoLineofText {
[cmdletbinding()]
param (
[parameter(HelpMessage = "Input of Array", Mandatory = $true)][array]$TheArray
)
$Line = ""
$TheArray = $TheArray | Sort-Object
foreach ($Info in $TheArray) {
$Line += """$Info"","
}
$Line = $Line.Substring(0, $Line.Length - 1)
$Line | Clip
Write-Host "Input is on in your clipboard: $Line"
}
Roadmap
Like all things, this can be improved upon as well. Here are some ideas and possible future growth.
- Validation that the input object is an array
- validation that the input is a single object array instead of a multi object array.
- A way to handle multiple layers of an array.
Thank you for reading, if you have any questions, feel free to contact me.