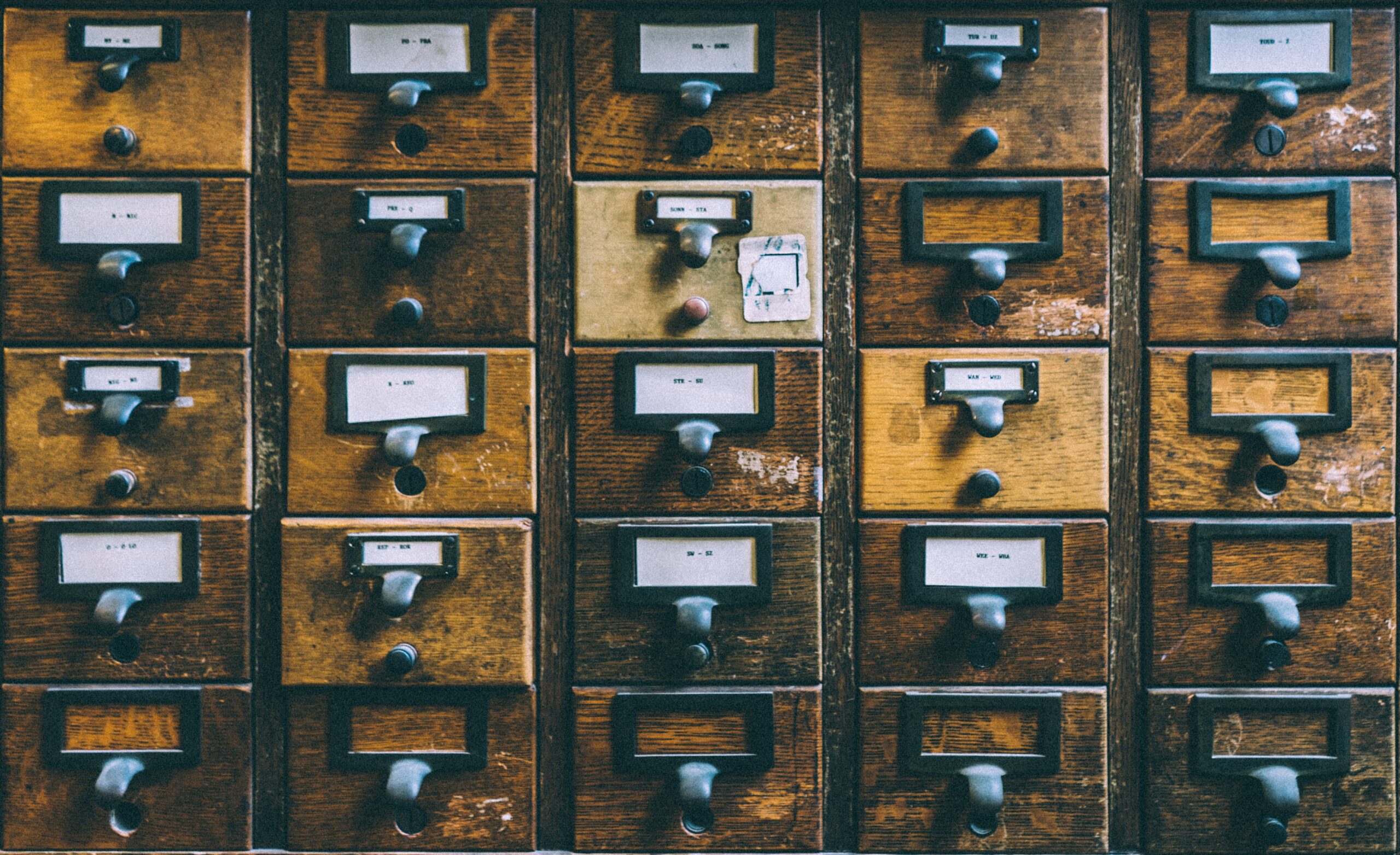
Exchange Online – Mailbox Size Audit
Here is a little powerhouse script I wrote to audit the mailbox sizes. The focus recently is to see who’s mailbox sizes are about to be over and if it’s the deleted folder. I can’t show you all of the scripts, but I can show you part of it. This part will pull the data down in such a way that you can view the mailbox sizes, who has the largest mailboxes, what folder is the largest in those mailboxes, and what their deleted folder is sitting at. Let us take a look at the script itself.
The Script
function Get-SHDEXOMailboxSizeAudit {
[cmdletbinding()]
param (
[pscredential]$Credential
)
Begin {
#Installs required modules
Write-Verbose "Installing required modules"
if (!(Get-InstallEdModule ExchangeOnlineManagement)) { Install-Module ExchangeOnlineManagement }
Write-Verbose "Checking and importing required modules"
# Starts importanting required modules
if (!(Get-Command Connect-ExchangeOnline)) { Import-Module ExchangeOnlineManagement }
}
process {
#Connecting to exchange. Grabing credentials.
if (!($PSBoundParameters.ContainsKey('Credential'))) {
$Credential = (Get-Credential)
}
Connect-ExchangeOnline -credential $Credential
#Grabs all the mailboxes at once. As this report will look at all the mailboxes.
$Mailboxes = Get-Mailbox
#Starts looping through each mailbox
For ($M = 0; $M -le $Mailboxes.count; $M++) {
Write-Verbose "Gathering Info on: $($Mailboxes[$M].UserPrincipalName)"
#Grabs the mailbox stats.
$Stats = Get-EXOMailboxStatistics $mailboxes[$M].UserPrincipalName
$FolderStats = Get-EXOMailboxFolderStatistics $mailboxes[$M].UserPrincipalName
#Starts looping through those folders to get their sizes.
$MainFolderStats = foreach ($Folder in $FolderStats) {
$FolderSize = [math]::Round((($Folder.FolderSize.split('(')[1].split(' ')[0] -replace ',', '') / 1gb), 2)
[pscustomobject]@{
Name = $Folder.name
Size = $FolderSize
}
}
#Adds this information to the mailbox object
$Mailboxes[$M] | add-member -Name "Statistics" -MemberType NoteProperty -Value $Stats
$Mailboxes[$M] | add-member -Name "FolderStats" -MemberType NoteProperty -Value $MainFolderStats
}
#Creates a return value.
$Return = foreach ($Mail in $Mailboxes) {
#Starts looking at mailboxes that are not the discovery mailbox.
if (!($mail.UserPrincipalName -like "DiscoverySearchMailbox*")) {
#Grabs the deleted folder as that is a folder we want to see in this report.
$Deleted = $Mail.FolderStats | where-object { $_.name -like "Deleted Items" }
#Grabs the largest folder. If it's not the deleted folder, then we might want to increase their mailbox sizes.
$LargestFolder = $Mail.FolderStats | Sort-Object Size -Descending | select-object -first 1
#Doing some math on a string. The string format (# bytes). Thus, we work the string to get the bytes. Divide and round.
$Size = [math]::Round(($Mail.Statistics.TotalItemSize.value.tostring().split('(')[1].split(' ')[0].replace(',', '') / 1gb), 2)
#Grabs the mailboxes percentage.
$DeletedToMailboxPercent = [math]::Round((($Deleted.Size / $size) * 100), 0)
#Outputs the data to the return value.
[pscustomobject]@{
DisplayName = $Mail.Displayname
UserPrincipalName = $Mail.UserPrincipalName
MailboxSize = $Size
LargetsFolder = $LargestFolder.Name
LargetsFolderSize = $LargestFolder.Size
DeletedItemSize = $Deleted.Size
DeletedToMailboxPercent = $DeletedToMailboxPercent
}
}
}
#Disconnects exchange
Disconnect-ExchangeOnline -confirm:$false > $null
}
End {
$Return | sort-object MailboxSize -Descending
}
}
The breakdown
First this script is designed to work on powershell 7. It will not work on powershell 5.
The first part we come to is the [pscredential] object. Notice it’s not mandatory. Notice no pipelining either. I have found PS credentials pipped intend to do very poorly. So, it’s simple, bam wam done.
Begin
Inside our begin tab, we have the module setup. We check installed modules for exchangeonlinemanagement. if it’s there, we ignore it and import the module, if it’s not we install it. Same way with importing. If it’s imported, then we do nothing, if it’s not, we import it.
Process
Next, we grab credentials if need be and connect to exchange. We use the get-credential command to grab the credentials. Then we use the connect-exchangeonline command to connect to the exchange online.
Once we are connected, the magic can start. This is the sweetness of this script. The first step is to grab all of the mailboxes at once with Get-Mailbox. Then we start a loop, not any loop, a for a loop. Wait! David, WHY A FOR LOOP! It’s simple, we want to add information to the index. So, we need to be able to call the index. We use the Get-EXOMailboxStatistics and choose the userprincipalname of the index we are looking for. We do the same thing with Get-EXOMailboxFolderStatistics. These gives us some clear stats we can use later on in the script. Now we loop through the folder stats that we just collected and math ourselves some bytes to gb. See the output of the get-exomailboxfolderstatistics looks like “24mb (#### bytes). So we need to filter that out and get the ####. I use a split to do this. I split out the string at the ‘(‘. This way, the bytes are on object 1. Then we split it again by the space. Now the bytes are on the 0 object. Then we replace the ‘,’ with nothing. Now we divide all that by 1gb to convert it to gbs. Then we drop that all into the mainfolderstats. Next, we add all of that information into the mailbox variable we created before this looping madness using add-member. We are adding it as a noteproperty.
Now we have prepped our data, it’s time to sort it. We start the loop once more, but this time it’s simple for each loop as we don’t need the array index value. We first grab all the deleted items folder. Then we grab the largest folder using the sort-object command on the size object of the folderstats note property that we made in the last step. Then we do some math. Like before to get the mailbox overall size. Finally, we grab the deleted mailbox percentage with a little more math. This time its percentage math. Now we have all of this useful information we use our pscustomobject and put it all together.
Then we disconnect using the disconnect-exchangeonline command.
End
Finally we display the return information inside our end tab. We sort the object by the mailbox size in a descending order.