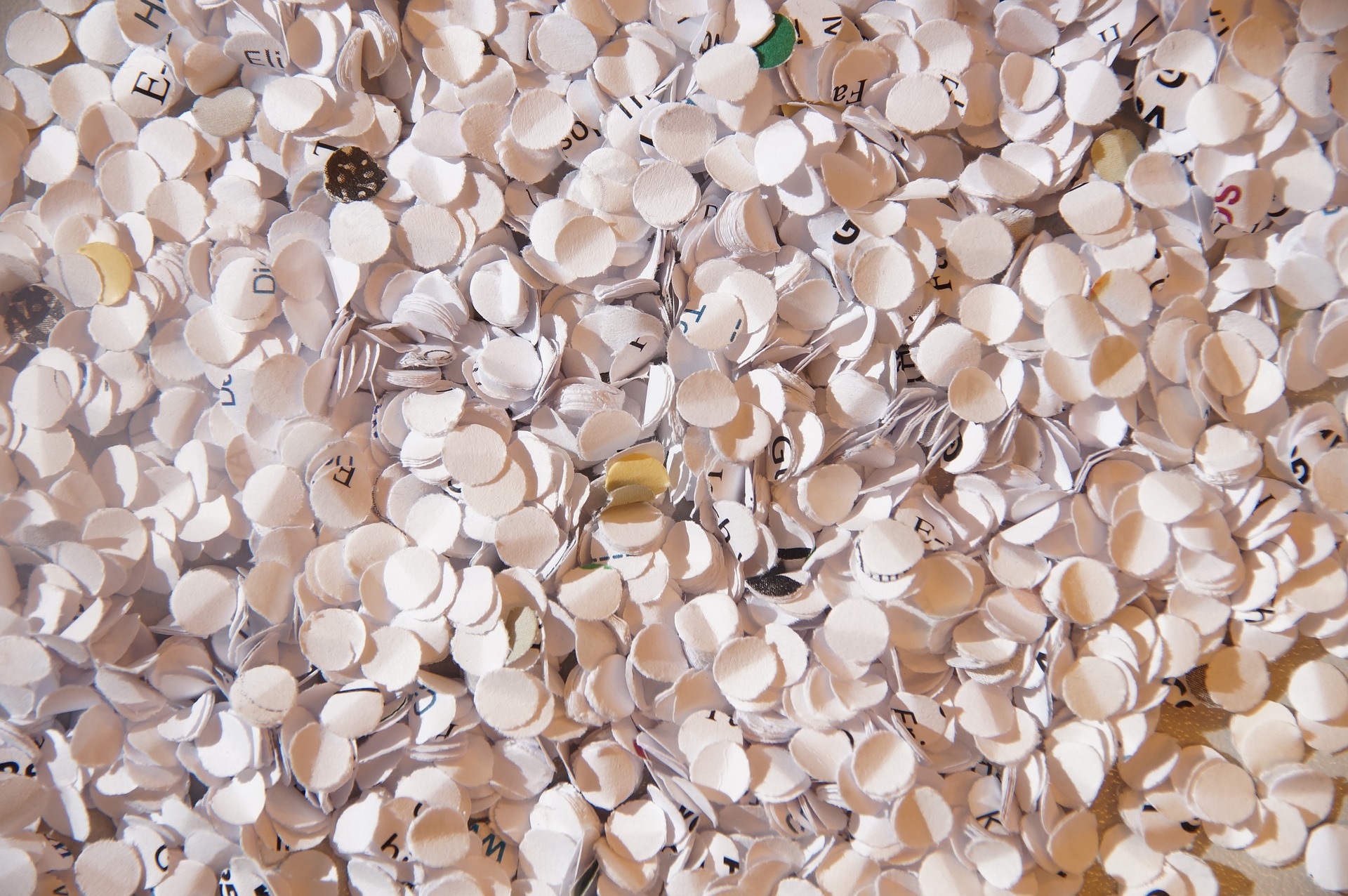
Test a Registry Value with PowerShell
The other day I needed to test if a registry key was present on an end user’s computer and make it if it didn’t exist. I performed a registry key value test with PowerShell. Since I was doing more than one, I pulled an older tool from my tool box for this one. It’s small but easy to use.
The Script
function Test-RegistryValue {
param (
[parameter(Mandatory = $true)][ValidateNotNullOrEmpty()][string]$Path,
[parameter(Mandatory = $true)][ValidateNotNullOrEmpty()][string]$Value,
[switch]$ShowValue
)
try {
$Values = Get-ItemProperty -Path $Path | select-object -ExpandProperty $Value -ErrorAction Stop
if ($ShowValue) {
$Values
}
else {
$true
}
}
catch {
$false
}
}
The Breakdown
This script is only a “Try Catch” with some added inputs. We are first grabbing what we want to test in our parameters. We have two mandatory strings and a switch. The first string is for the path in which we are going to test. The second is the value we want to test. for example, if we want to see if google earth has a version number, we would give the path of HKLM:\Software\Google\Google Earth Pro and the value of Version. If we want to see that version we flip the next part which is the only switch, show value. Instead of saying true or false it will say the value.
Try Catch
$Values = Get-ItemProperty -Path $Path | select-object -ExpandProperty $Value -ErrorAction Stop
Inside our try-catch box, we are using the Get-ItemProperty command. We select the $Value. Finally, we stop the command using the error action flag stop. This prevents the command from falling apart. Next, we throw all that information into the $Values parameter.
try {
$Values = Get-ItemProperty -Path $Path | select-object -ExpandProperty $Value -ErrorAction Stop
if ($ShowValue) {
$Values
} else {
$true
}
} catch {
$false
}
Aftward, we use a basic if statement. We show the value when the “showvalue” flag is set. However, if it’s not, we just send a true. Finally, the catch will tell us a false statement if the Get-ItemProperty command failed for any reason.
Conclusion
There are other ways to do this, but this was the quickest I have found. I added the show Value recently because I needed it for troubleshooting the code. Overall, this little guy is perfect to add to any script that deals with registry changes.