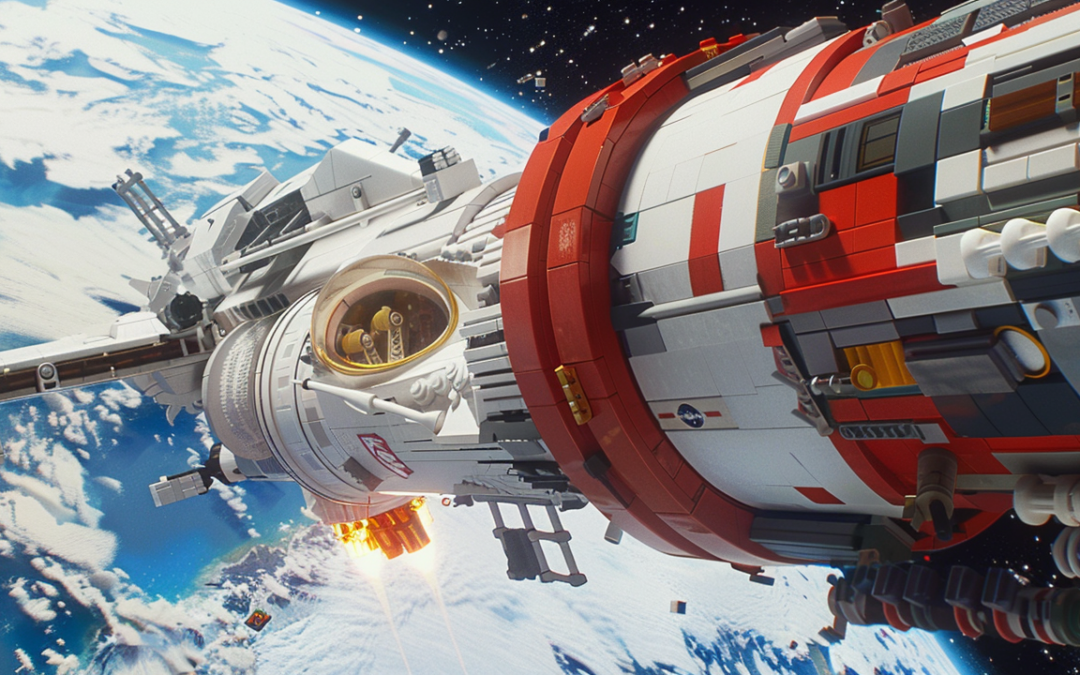
PowerShell script block tutorial
Here, we’ll learn how to use the & operator to run script blocks—your go-to PowerShell script block tutorial! You’re not alone if you’ve ever thought PowerShell scripting was a little confusing. Today, we’re simplifying the & operator, one of PowerShell’s most important features, and making it really easy to use. Knowing how to use this operator successfully will improve your scripting talents, regardless of experience level. Together, we can make writing scripts as pleasurable as indulging in your preferred treat after a demanding day! Join me as we dive into our PowerShell script block tutorial.
What is the &
Operator?
Your key to running commands kept in script blocks or variables in PowerShell is the & operator, sometimes referred to as the call operator. Consider it as your script’s magic wand, waved to bring it to life. What use is this to us? As simple as entering Write-Host “Hello, World!” commands aren’t always clear-cut. The & operator exists to make sure things go smoothly and precisely as intended when you start storing commands in variables or need to perform complicated expressions kept as script blocks.
For example, typing just $command in PowerShell will return the string “Get-Date” if your command is $command = “Get-Date”—not very useful if you’re trying to find out the time! But & $cmd runs it, converting your string into a useful command. That seems easy enough, right? Still, so potent.
Understanding Script Blocks
Moving deeper into the rabbit hole, let’s talk about script blocks. In PowerShell, a script block is essentially a collection of statements or expressions that are written as a single unit but executed as needed. Imagine them as your script’s building blocks, where each block is crafted to perform specific tasks.
Syntax-wise, a script block is encased in curly braces {}
. Here’s a straightforward example:
$myScriptBlock = {
param ($name)
"Hello, $name! Today is $(Get-Date)."
}
In this script block, we’re passing a parameter $name
and outputting a friendly greeting along with the current date. But without our trusty &
operator, this friendly message remains locked inside its curly brace prison. By calling & $myScriptBlock -name 'Frank'
, you breathe life into it, and out pops, “Hello, Frank! Today is [current date].”
Script blocks can be as simple or as complex as you need them to be, handling anything from quick one-liners to extensive scripts requiring loops, conditionals, and more. They’re incredibly powerful because they let you neatly package and repeatedly execute chunks of code with minimal fuss.
Executing Script Blocks with the &
Operator
Now, let’s get our hands dirty and see the &
operator in action. We’ll take the script block we mentioned earlier and really break it down. This script block scrambles a message—a fun way to see the power of randomization in PowerShell:
$myScriptBlock = {
param ($msg)
$charArray = $msg.ToCharArray()
$random = New-Object System.Random
[Array]::Sort($charArray, [System.Collections.Generic.Comparer[char]]::Create({param($x,$y) $random.Next(-1,2)}))
$scrambledMsg = -join $charArray
Write-Host "$scrambledMsg"
}
To execute this script block and see your message get all mixed up, you’d use:
& $myScriptBlock "I like cheese cake on my chocolate syrup."
This line invokes the script block with the &
operator and passes the string “I like cheese cake on my chocolate syrup” as an argument. The script takes each character, scrambles them, and outputs something that looks like your original message went through a blender. It’s a practical, hands-on way to see script blocks and the &
operator in perfect harmony.
Practical Examples
While our scrambled message is fun, let’s look at more practical uses of script blocks and the &
operator in everyday scripting tasks. Here are a few scenarios:
- Batch Renaming Files:This script block takes a file and a new name as parameters and renames the file accordingly.
$files = Get-ChildItem -Path "C:\MyDocuments" $renameScript = { param ($file, $newName) Rename-Item $file.FullName -NewName $newName } foreach ($file in $files) { & $renameScript $file "New_$($file.Name)" }
- Processing Log Files:
$processLog = { param ($logPath) $logContents = Get-Content $logPath $logContents | Where-Object { $_ -match "ERROR" } | Set-Content "FilteredErrors.log" } & $processLog "C:\Logs\server.log"
Advanced Tips and Tricks
To elevate your scripting game, here are some advanced tips and tricks for using script blocks and the &
operator in PowerShell:
- Passing Multiple Parameters: You can pass multiple parameters to a script block by simply separating them with commas after the script block call:
& $myBlockParam $arg1, $arg2, $arg3
- Using Script Blocks for Asynchronous Tasks: PowerShell allows script blocks to be run asynchronously, which can be great for performance when dealing with long-running tasks
$job = Start-Job -ScriptBlock $myScriptBlock -ArgumentList "ParamValue"
- Error Handling within Script Blocks: Always include error handling within your script blocks to manage exceptions smoothly
$errorHandlingBlock = { try { # Potentially risky operations } catch { Write-Error "Something went wrong!" } } & $errorHandlingBlock
Wrapping Up
Congratulations for delving deeply into the operator and script blocks of PowerShell! We’ve unpacked a lot of really technical material today, transforming what may have appeared like magic into a set of useful abilities you can apply immediately. We began with a review of the & operator, looked at defining and running script blocks, and even played about with message scrambling. We next advanced to increasingly difficult scenarios to show how script blocks might make chores like batch renaming files or sorting through logs easier.
Writing more effective and efficient scripts is easy when you have the & operator in your toolbox. PowerShell is a potent tool. Recall that putting in constant practice is the best approach to improve. Try out several script blocks, modify our examples, and learn how you might use them for everyday chores.
For those who are itching for more, I suggest you to explore more sophisticated PowerShell scripting methods or sign up for PowerShell forums and groups. There is always a new, clever method to learn in the field of scripting, and learning never really ends.
May your scripts always run well and happy scripting! Thank you for looking at our PowerShell script block tutorial.
What can we learn as a Person?
Here’s the thing about PowerShell script blocks: they’re modular. You write them once and use them wherever needed without starting from scratch each time. It’s neat, tidy, and incredibly efficient. Now, let’s take this concept and apply it to something a bit closer to our everyday lives—our work-life balance.
Why Boundaries Matter
Imagine your life as a complex script. Each segment—work, family, personal time—is like a little block of code. Just like in scripting, you don’t want these blocks interfering with each other more than they need to. It messes things up. If work starts to bleed into family time regularly, it’s like a script executing commands where it shouldn’t. Before you know it, you’re looking at a full-blown system crash (or in human terms, a burnout).
Recycling What Works
Why reinvent the wheel? If you’ve nailed a routine that keeps your work and personal life neatly compartmentalized, keep that going. Apply it as often as needed, just like a reusable script block. Found that turning off email notifications after 6 PM helps keep your evenings calm? Make it a staple. Effective routines are like code that doesn’t need debugging—they just work, and they save you a ton of mental energy.
Tweaking the System
Life, just like software, updates and changes all the time. New job? Family dynamics shifted? Just as a programmer tweaks a script to fit new requirements, you might need to adjust your boundaries and routines to keep everything running smoothly. It’s not about sticking rigidly to old scripts but adapting them to better fit your current scenario.
So, let’s take a leaf out of our script book. Setting clear, modular boundaries in our lives isn’t just about keeping things orderly. It’s about ensuring we don’t run ourselves into the ground. After all, nobody’s got time for downtime, right?