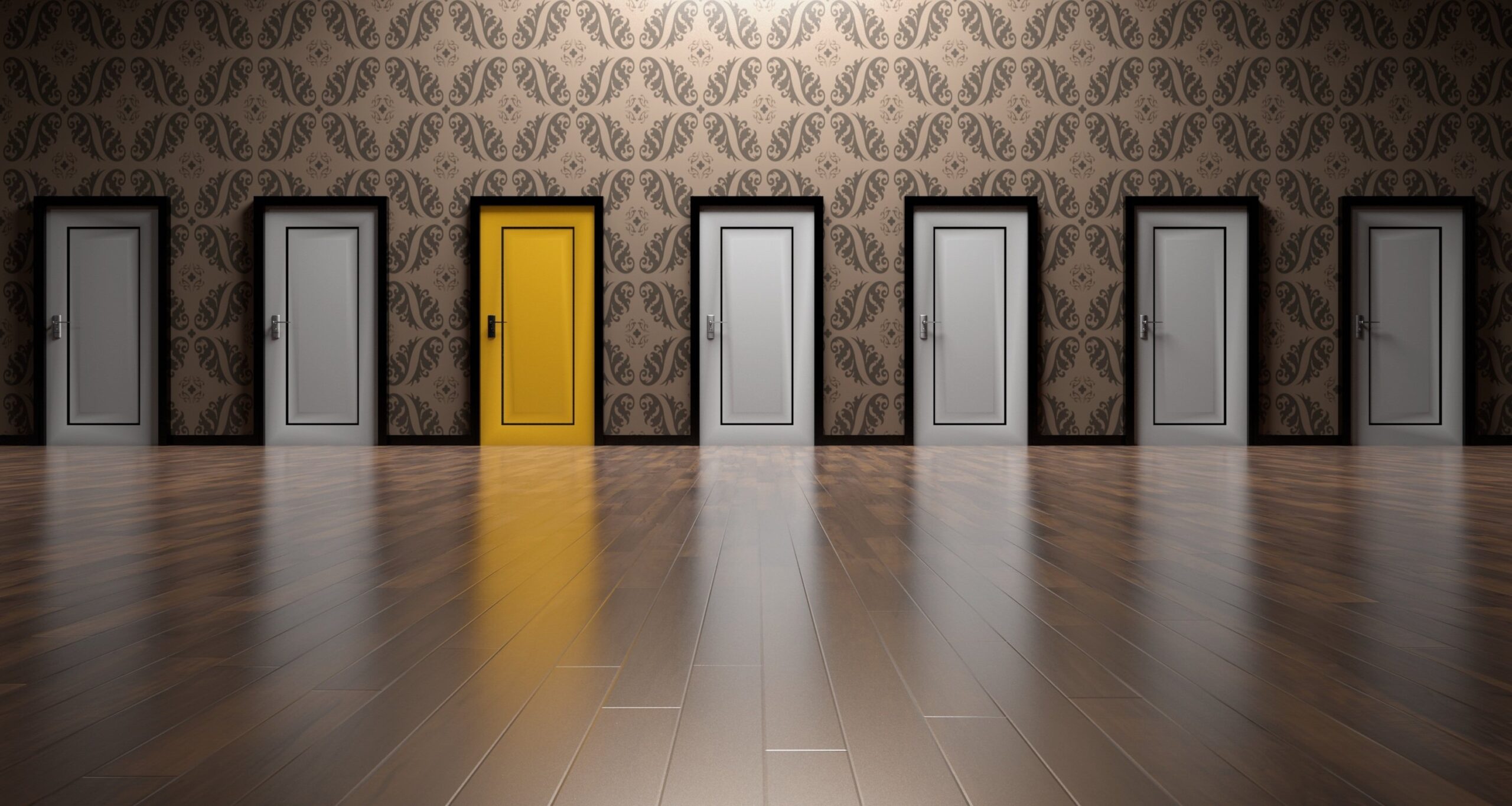
Ping a /24 Subnet with Powershell
A friend asked me how to ping a /24 subnet the other day. I thought it would be a good little blog post. This is a one-liner. Here we go.
1..254 | ForEach-Object {Test-Connection -ComputerName "10.10.1.$_" -Count 1 | Select-Object Source,Destination,Address,Status} | Format-Table -autosize
Let’s break this guy down. The 1..254 basically is every number between 1 and 254. We pipe that into a foreach-object loop. This will repeat whatever is inside the loop. In this case 254 times. Inside the loop, we use the Test-Connection command and set up the -ComputerName flag. Next, we set the subnet that we want to explore. Here it’s “10.10.1.$_” The $_ means the pipped object. The first run through the computer name will be “10.10.1.1” and so on. The next flag is the -count flag. This is how many times you want to try to connect to the device. I set it to 1 because the default is 3. Making it one is much faster and less confusing. Then I pipe that data into the select-object command. I select the source, destination, address, and status of the command. This gives me a clear and less confusing picture of everything. Then we close up the loop. Right here we can be finished. Sometimes though, Powershell’s shell is a pain the arse. The outlook would start looking like the this:
Lap-01582 10.10.1.97 TimedOut
Lap-01582 10.10.1.98 10.10.1.… Success
Lap-01582 10.10.1.99 10.10.1.… Success
Lap-01582 10.10.1.1… 10.10.1.… Success
Lap-01582 10.10.1.1… 10.10.1.… Success
Lap-01582 10.10.1.1… 10.10.1.… Success
Lap-01582 10.10.1.1… 10.10.1.… Success
To prevent this, we can use the Format-Table command with the -autosize flag. This will increase the size so it’s easier to read the information. The downside is it takes longer to get the feedback as you are piping all that information into a single command. Another thing you can do is export the information into a csv by using the export-csv command.
1..254 | ForEach-Object {Test-Connection -ComputerName "10.10.1.$_" -Count 1 | Select-Object Source,Destination,Address,Status} | export-csv -Path "$Env:USERPROFILE\Desktop\pingreport.csv" -NoClobber
This export command gives you the ability to place it wherever you want. I like it to go to either my desktop or my documents. So I use the $env:Userprofile variable. This Variable will give the shell’s current user’s profile and where it lives. Then you just tell it where to go after that. Notice I use the -NoClobber flag. This flag tells the export-csv to not add a line at the beginning of the csv stating it was generated by Powershell. Now I have a spreadsheet of this ping report.
I hope this helps, as always, if you need help, feel free to reach out.