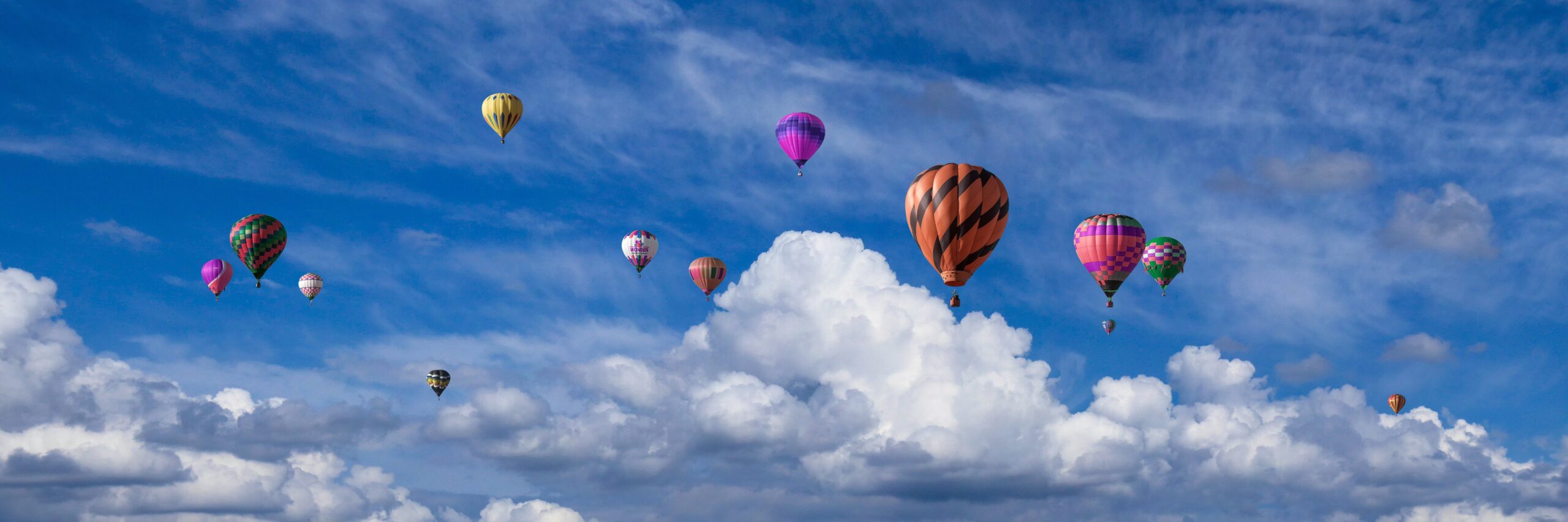
Message in The Ballon
Do you need something to let your end-user know what’s going on? Don’t want your end-user to see the program running? Well, PowerShell has the ability to use the system windows notify system. AKA, the message box that pops up on the right of the screen.
The first step to make this happen is we need to add the assembly, system.windows.forms. We do this by the amazing command add-type.
add-Type -AssemblyName System.Windows.Forms
Now we have the assemblies loaded into ram, we need to create an object from those assemblies. This way we can command the object later. We do this using the New-object cmdlet. We want the notification tray icons. So we want the system.windows.forms.notifyicon.
$global:balloon = New-Object System.Windows.Forms.NotifyIcon
You know that notifications have icons. Yep, It’s from the application itself. So, if you have this function in another application, it will show that the application’s icon. We call upon the System.Drawing.Icon to do this. But First, we need to know which app we are currently using. We do this with Get-Process with the ID and the current Pid.
$path = (Get-Process -id $pid).Path
$balloon.Icon = [System.Drawing.Icon]::ExtractAssociatedIcon($path)
What’s cool about this is we are extracting the icon of the application itself with that extract associated icon. We push it into our notify icon object. Next, we want to add the Tip Icon. That’s the ! ? so on and so forth. We do this by using the system.windows.forms.tooltipicon function. Believe it or not, we can use a validate set in the parameters to get the exact naming input as well. The property of the object is BalloonTipIcon.
$balloon.BalloonTipIcon = [System.Windows.Forms.ToolTipIcon]::$Messagetype
Now the message itself. At this point we have an icon, the notification icon and that’s it. This is the meat of the notification. The propriety in the balloon object is, BalloonTipText.
$balloon.BalloonTipText = $Message
Just a quick note, to much text is ugly.
Next is the title, I like to use just the word attention as it brings the users eyes to it. The balloon’s propriety for this is BalloonTipTitle.
$balloon.BalloonTipTitle = "Attention"
Finally, we want to make this thing visible. An invisible tooltip is as useful as invisible ink without a black light. As you have guessed by now, Visible is the balloon’s propriety.
$balloon.Visible = $true
Now the drum roll, please! It’s time to show our masterpiece that will be enjoyed by the end-user for a split second. The ShowBalloonTip(). Yes, let us do it! This is a method and will show the balloon tip for x time. This is set up in milliseconds. So, every 1000 milliseconds makes a second.
$balloon.ShowBalloonTip($Time)
Lets put it all together shall we. Behold the script of the balloon tip.
The Script
Function Invoke-SHDBallonTip {
[cmdletbinding()]
param (
[string]$Message = "Your task is finished",
[int]$Time = 10000,
[validateset("Info", "Warning", "Error")][string]$Messagetype = "Info"
)
#Adds the reqruired assembly's for the ballon tips.
Add-Type -AssemblyName System.Windows.Forms
#Creates the notifiy icon that will appear in the notification tray.
$global:balloon = New-Object System.Windows.Forms.NotifyIcon
$path = (Get-Process -id $pid).Path
$balloon.Icon = [System.Drawing.Icon]::ExtractAssociatedIcon($path)
$balloon.BalloonTipIcon = [System.Windows.Forms.ToolTipIcon]::$Messagetype
$balloon.BalloonTipText = $Message
$balloon.BalloonTipTitle = "Attention"
$balloon.Visible = $true
$balloon.ShowBalloonTip($Time)
}
That’s it, folks. Such a simple thing. Here are a few things you can do. You can change the $path to $Path = Get-Process -id $PID. Then in the balloon.icon, use $path.path. In the balloon tip title, you can put $path.description and now you have the description as the title. Other than that, it’s pretty straight forward.
I would love to hear back from yall. Let me know if there is anything you would like for me to write about.