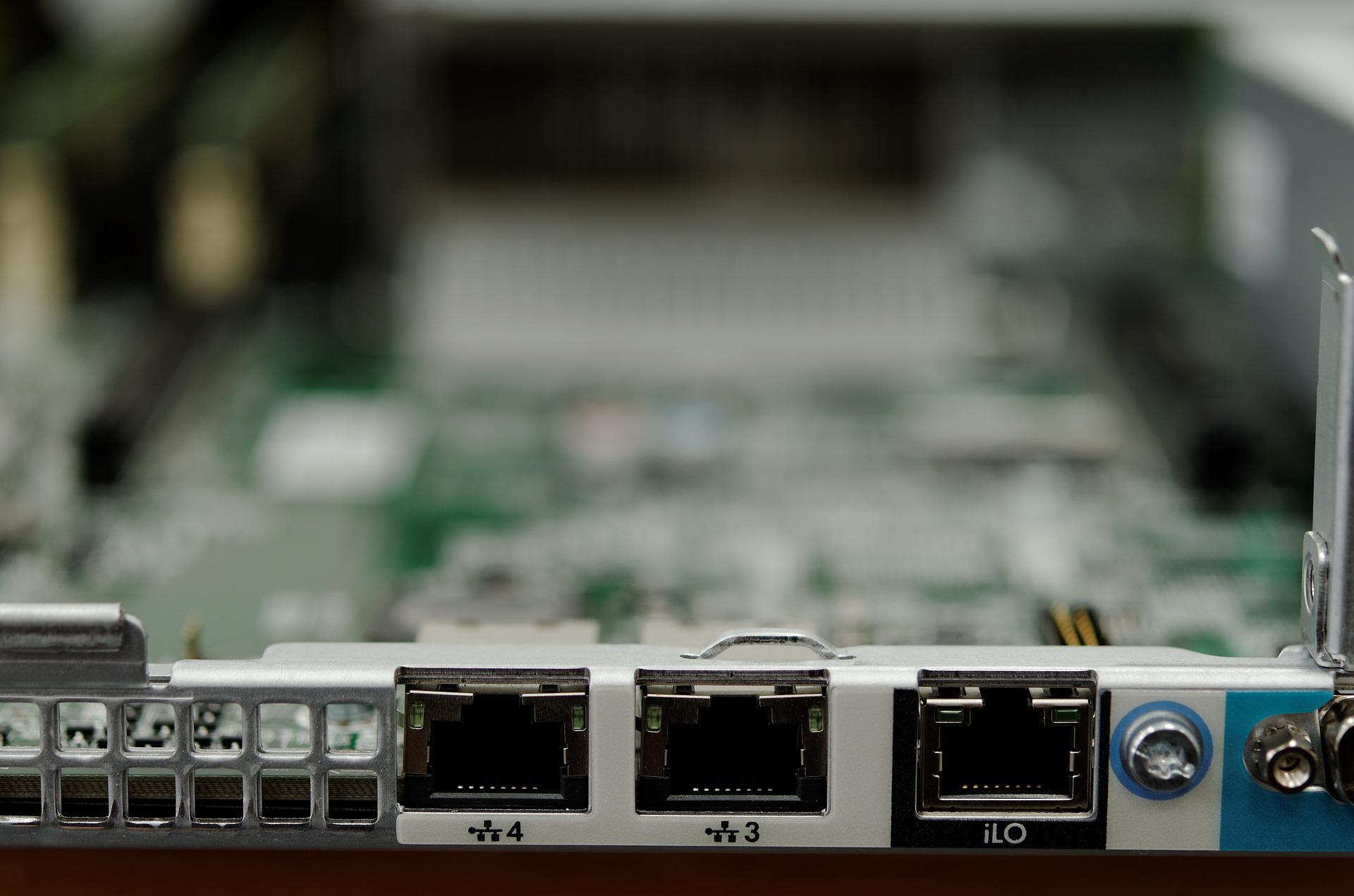
In the Field – IP and Mac Info
Today in the field, we needed to find all of the device’s IP and Mac Info for each network adapter. This was a large undertaking. We were looking for a special network adapter that didn’t show up in our normal reports from our RMM. However, our RMM could push out PowerShell scripts and return the network adapters for us. The information we needed was the network adapter index number. Then we needed the mac address and IP address. Finally, we needed the name of the network device. The stage is set.
Network Adapters
Firstly, we need to grab the network adapters. We are using the Get-NetAdapter command. This command lists all the current network adapters on the device in question.
$Adapters = Get-NetAdapter
The net adapter gives us the name of the adapter, the mac address, and the index we are looking for. Since we placed the net adapters in a workable variable, we can loop this variable. While looping, we are targeting the interface index.
Net IP Address
Secondly, the next piece we need is the IP information from each network adapter. We do this with the Get-NetIPAddress command. This command grabs the IP address of each network adapter, along with the interface Index. This means we can marry the mac address to the IP address. This command produces both the IPv4 and IPv6 address.
$IP = Get-NetIPAddress
This command produces a large list of useful information. The information we want is the index, ipv4 and ipv6 addresses. Since we have both pieces of the pie, it’s time to marry the two.
IP and Mac Info
As a result of having the above commands information, we can marry these two together. We start with a foreach loop of the adapters.
$Return = foreach ($Adapter in $Adapters) {
#Do Something
}
We are looping the adapters because it’s the physical side of things. Plus, the IP information directly links to the network adapter. We place the foreach loop information into a variable. This allows us to filter later. Next, is the PS custom object. A PS custom object is the object we are after.
$Return = foreach ($Adapter in $Adapters) {
[pscustomobject][ordered]@{
#Information
}
}
It’s within this object that magic happens. The current adapter will provide the name, interface description, mac address, index, link speed, and status. From there we will grab the IPv5 address by using where-objects. First, we search for the index of the IP command for the adapter index. Then we search for the address family to be IPv4. We repeat this process for IPv6. This is what the loop looks like.
$Return = foreach ($Adapter in $Adapters) {
[pscustomobject][ordered]@{
Name = $Adapter.Name
Description = $Adapter.InterfaceDescription
MacAddress = $adapter.MacAddress
InterfaceIndex = $Adapter.Interfaceindex
LinkSpeed = $Adapter.LinkSpeed
Status = $Adapter.Status
IPV4 = ($IP | where-object { $_.InterfaceIndex -eq $Adapter.Interfaceindex } | where-object { $_.addressfamily -like "IPv4" }).IPaddress
IPV6 = ($IP | where-object { $_.InterfaceIndex -eq $Adapter.Interfaceindex } | where-object { $_.addressfamily -like "IPv6" }).IPaddress
}
}
After that, the loop finishes and we display a return. We sort the object by the link status, this way we see the active ones first.
$Return | Sort-Object -Property Status
The Script – IP and Mac Info
$Adapters = Get-NetAdapter
$IP = Get-NetIPAddress
$Return = foreach ($Adapter in $Adapters) {
[pscustomobject][ordered]@{
Name = $Adapter.Name
Description = $Adapter.InterfaceDescription
MacAddress = $adapter.MacAddress
InterfaceIndex = $Adapter.Interfaceindex
LinkSpeed = $Adapter.LinkSpeed
Status = $Adapter.Status
IPV4 = ($IP | where-object { $_.InterfaceIndex -eq $Adapter.Interfaceindex } | where-object { $_.addressfamily -like "IPv4" }).IPaddress
IPV6 = ($IP | where-object { $_.InterfaceIndex -eq $Adapter.Interfaceindex } | where-object { $_.addressfamily -like "IPv6" }).IPaddress
}
}
$Return | Sort-Object -Property Status
Conclusion
Even though this case was a fringe case, it still shows the power that Powershell can provide. We were able to grab the IP and Mac Info for each network adapter. From there we were able to generate the report needed. Pull all of the Mac addresses and IP addresses needed to complete the project. Overall, this was a success for us. I hope you are able to use some if not all of this code somewhere.