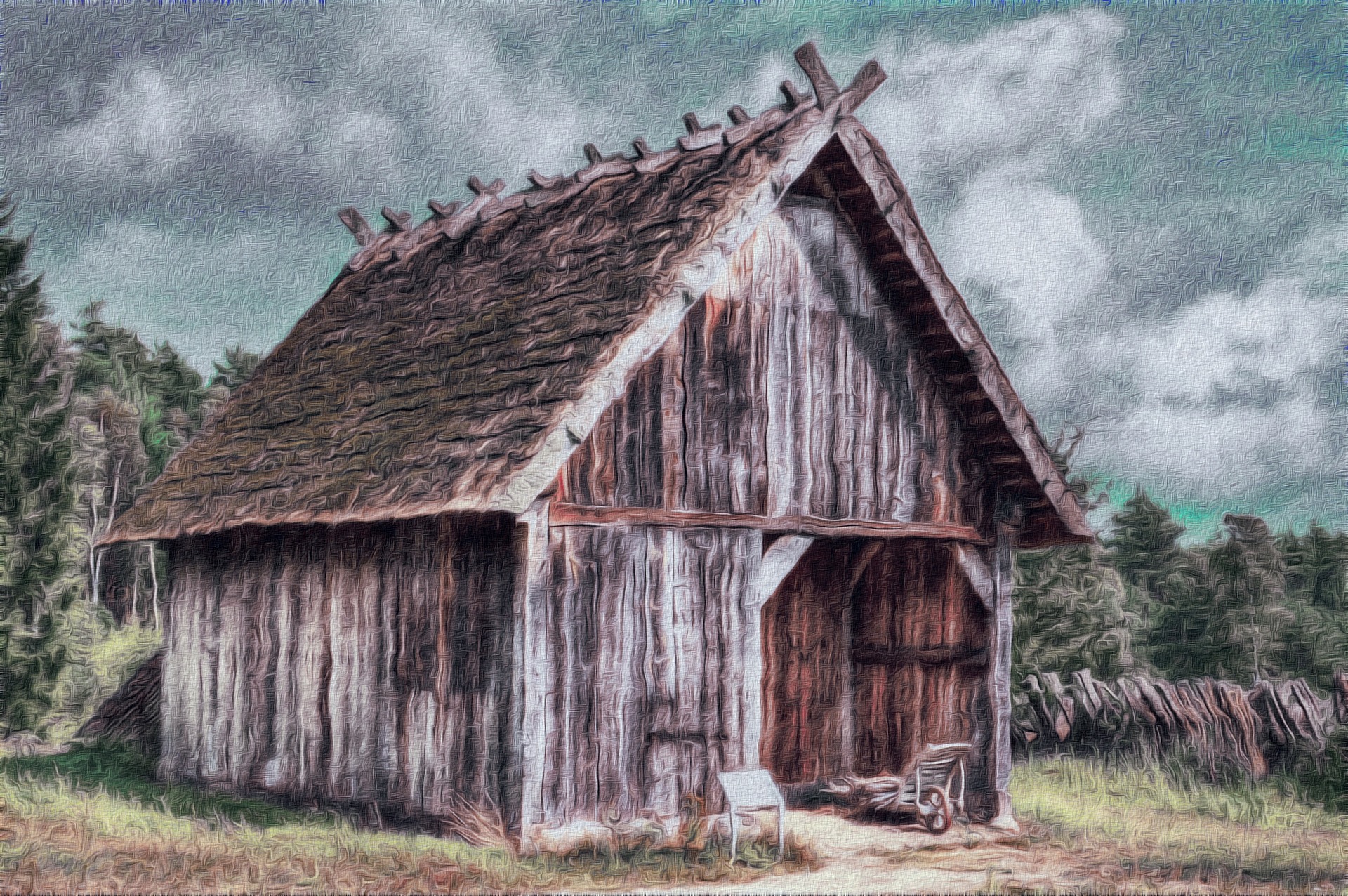
Install Gimp with Powershell
This little script installs the latest version of gimp 2.10 onto your Windows machine. Let’s take a look at the script and then break it down.
The Script
$DownloadPath = "C:\Temp\Gimp"
If (!(Test-Path -Path "C:\Temp\")) {New-Item -Path c:\ -Name Temp -ItemType Directory }
If (!(Test-Path -Path "C:\Temp\Gimp\")) {New-Item -Path c:\Temp -Name Gimp -ItemType Directory }
$URL = "https://download.gimp.org/mirror/pub/gimp/v2.10/windows/"
$Gimp = Invoke-WebRequest -UseBasicParsing -Uri $URL -SessionVariable websession
$Links = $Gimp.Links | Where-Object {$_.href -like "*.exe"} | select-object -Last 1
$URLDownload = "$URL$($Links.href)"
$DownloadName = "$DownloadPath\Gimp.exe"
Invoke-WebRequest -Uri $URLDownload -OutFile $DownloadName
if (Test-path "C:\Program Files\GIMP 2") {
Get-Process -Name "Gimp*" | Stop-Process
Start-Process -FilePath "C:\Program Files\GIMP 2\uninst\unins000.exe" -ArgumentList "/VERYSILENT" -wait
}
Start-Process -FilePath $DownloadName -ArgumentList '/VERYSILENT /NORESTART /ALLUSERS' -wait
Remove-Item $DownloadName
The Breakdown
The first thing we do is set up the path we want to make. Then we test to see if the path exists. If they don’t, we make them. I’m using temp in this cause because I will be deploying this to 2000+ machines. We will remove the installer afterward. I want the Temp folder to existing afterward for future deployments.
$DownloadPath = "C:\Temp\Gimp"
If (!(Test-Path -Path "C:\Temp\")) {New-Item -Path c:\ -Name Temp -ItemType Directory }
If (!(Test-Path -Path "C:\Temp\Gimp\")) {New-Item -Path c:\Temp -Name Gimp -ItemType Directory }
Next, we grab the URL we want to work with This is the gimp’s official download portal. This portal is by default Oldest to newest when you pull from it using Powershell.
$URL = "https://download.gimp.org/mirror/pub/gimp/v2.10/windows/"
Then we use the Invoke-webrequest to grab the website as we did in a previous post. From there we grab all of the links. In this case, since it’s a repo, they are all download links except for 2. We only want the exes of the list, so we use a where-object to find those. Then we select the last 1 as it is the newest version.
$Gimp = Invoke-WebRequest -UseBasicParsing -Uri $URL -SessionVariable websession
$Links = $Gimp.Links | Where-Object {$_.href -like "*.exe"} | select-object -Last 1
Now we need to build our URL and our Path. This is some string controls. Notice the $($Something.Something) in this code. When you deal with an array in a string and want to grab a sub item, you need to call it out with the $().
$URLDownload = "$URL$($Links.href)"
$DownloadName = "$DownloadPath\Gimp.exe"
Next we download the Gimp 2.10 version we are wanting with another invoke-webrequest. This time we select the Outfile tab.
Invoke-WebRequest -Uri $URLDownload -OutFile $DownloadName
Now we want to uninstall the pervious version of Gimp. Since gimp doesn’t show up in the win32_products, we go to it manually in the file system. Newer gimps host themselves inside the program files > gimp 2. So we search to see if that folder exists with a test-path. If it does, we then check to see if gimp is running. Then kill it with fire… ok, not fire, but force. Gimp is awesome about putting an uninstaller inside the file system. So we will use that. It’s located in the Gimp 2 > Uninst > Unins000.exe. Which can be triggered with a /verysilent parameter to keep it quiet. We do this with a start process and we use a flag -wait to wait on it to uninstall.
if (Test-path "C:\Program Files\GIMP 2") {
Get-Process -Name "Gimp*" | Stop-Process -Force
Start-Process -FilePath "C:\Program Files\GIMP 2\uninst\unins000.exe" -ArgumentList "/VERYSILENT" -Wait
}
Then we start the install of the new gimp with the start-process again. We use the Download Name we made eailer with an argument list of /verysilent /norestart /allusers and a -wait.
Start-Process -FilePath $DownloadName -ArgumentList '/VERYSILENT /NORESTART /ALLUSERS' -Wait
Finally we remove the installer with a remote-item.
Remove-Item $DownloadName
That’s all it takes yall. I hope this is helpful to you.