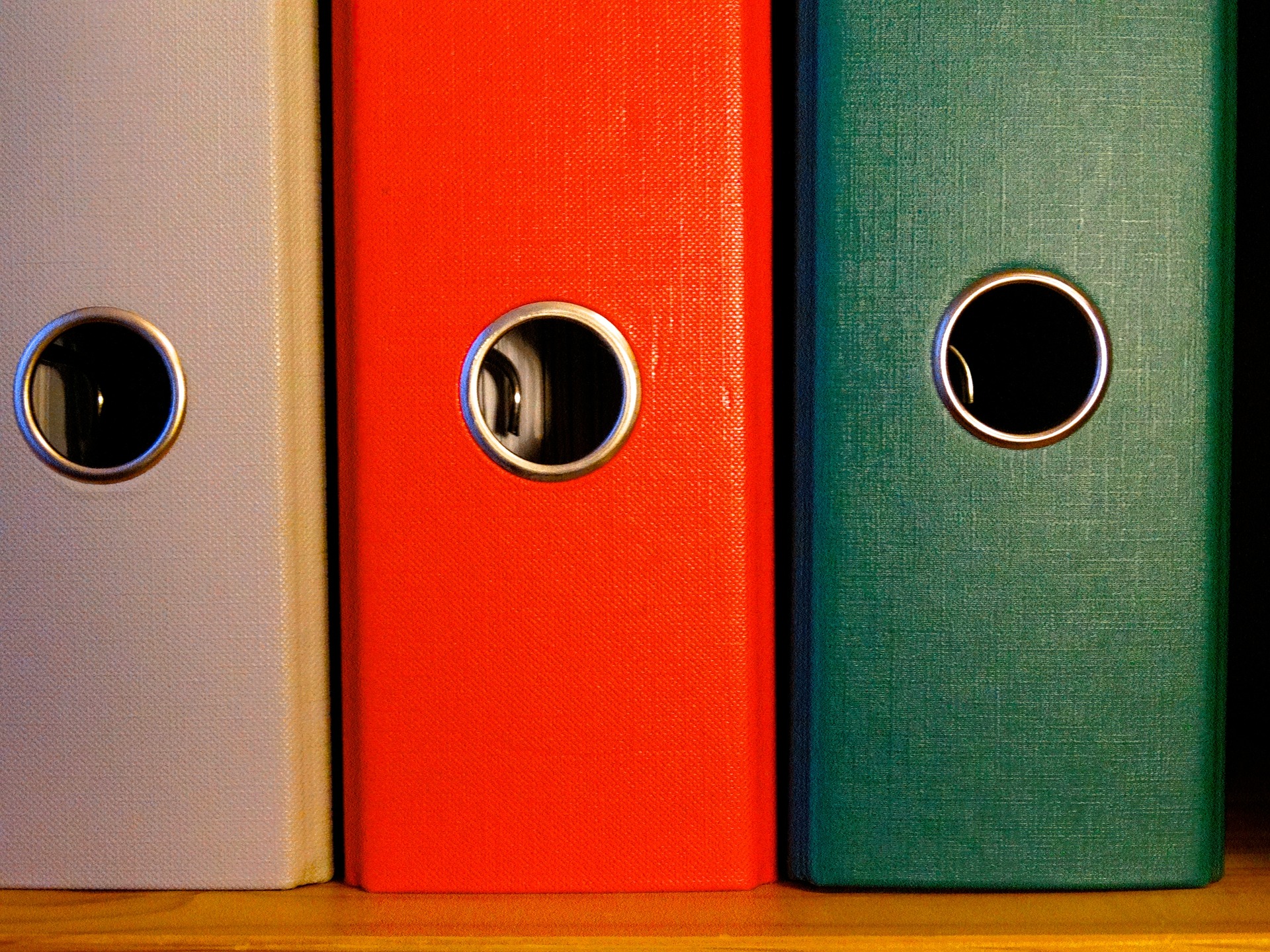
Resource – SHD Get ACL
Ever need to combine Get-childitem and Get-ACL while only pulling the access information and users? Well, here we are. I hope you all can use it well.
function Get-SHDACL {
[cmdletbinding()]
param (
[parameter(Mandatory = $true)][string]$Path,
[string]$Filter,
[switch]$Recurse,
[switch]$Directory,
[switch]$File
)
begin {
if ($PSBoundParameters.ContainsKey("filter")) {
if ($File) {
if ($Directory) {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -Directory -File -Filter $Filter
} else {
$SubPath = Get-ChildItem -Path $Path -Directory -File -Filter $Filter
}
} else {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -File -Filter $Filter
} else {
$SubPath = Get-ChildItem -Path $Path -File -Filter $Filter
}
}
} else {
if ($Directory) {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -Directory -filter $Filter
} else {
$SubPath = Get-ChildItem -Path $Path -Directory -Filter $Filter
}
} else {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -Filter $Filter
} else {
$SubPath = Get-ChildItem -Path $Path -Filter $Filter
}
}
}
} else {
if ($File) {
if ($Directory) {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -Directory -File
} else {
$SubPath = Get-ChildItem -Path $Path -Directory -File
}
} else {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -File
} else {
$SubPath = Get-ChildItem -Path $Path -File
}
}
} else {
if ($Directory) {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse -Directory
} else {
$SubPath = Get-ChildItem -Path $Path -Directory
}
} else {
if ($Recurse) {
$SubPath = Get-ChildItem -Path $Path -Recurse
} else {
$SubPath = Get-ChildItem -Path $Path
}
}
}
}
}
Process {
foreach ($Sub in $SubPath) {
$ACLinfo = Get-Acl -Path $sub.FullName
$Return += foreach ($ACL in $ACLinfo.Access) {
[pscustomobject]@{
Path = $Sub.FullName
FileSystemRights = $ACL.FileSystemRights
ID = $ACL.IdentityReference
Type = $ACL.AccessControlType
Inherited = $ACL.IsInherited
}
}
}
}
end {
$Return
}
}