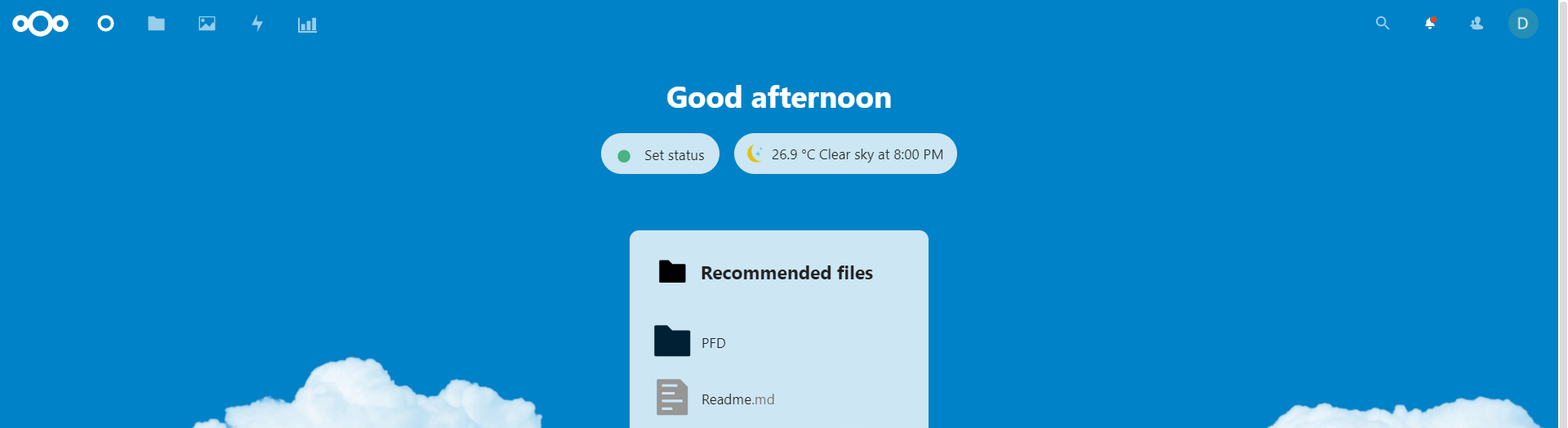
Next Cloud – File Drop
I love my nextcloud. It is hosted at my house, and I can upload files from anywhere in the world. It’s very clean, and one of the most awesome features is the file drop. I use scripts to upload the results all the time to the file drop location on my next cloud. A file drop is an upload-only file location. This means the outside world can not see the files inside of it. Only I can do that. I can also password lock this file drop location so only those with the password can get into it. It’s pretty cool.
How to setup a File Drop
I am going to assume you have a nextcloud. Once you log in, click the + icon to create a folder. We will call it PFD for password file drop and click the arrow to create.
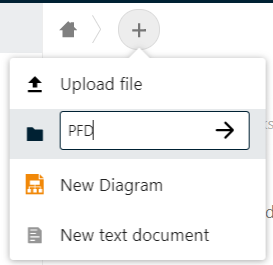
Now we will set up the sharing side with the password lock.
- Click the PFD folder to open the menu on the right hand side.
- Click the sharing icon
- Click the share link +.
- Click the three dots
- Radio check the File Drop (Upload Only)
- Check the Password Protect
- It will auto generate a password, It’s best to make your own.
- Document your password!
- Click the arrow to save the password
- Click the clip board to copy the link for your new share
- In a different browser test your link.
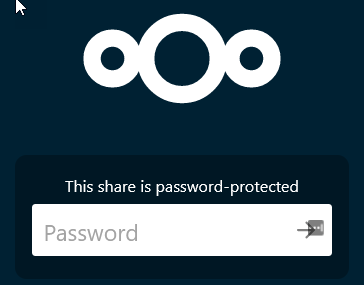
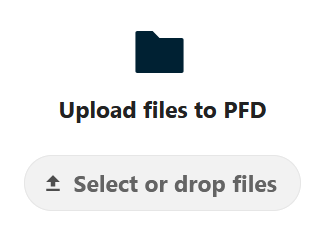
Now you have a safe place for the files to go. It’s time to upload files via PowerShell. You will need a few items. First the URL of your site. example https:\\cloud.bolding.us. Next, you will need the shareID of the folder in question. That can be found in your URL at the end. Example
- The URL. For this example we will be using https://cloud.bolding.us
- The shareID of the directory in question. It is the last part of the main url that we copied by clicking the clip board. I have bolded it for you in this example: https://cloud.bolding.us/index.php/s/oWHeW4dfWnxwXXX
- Next you will need the password.
Those are the three things you will need to create your invoke-restmethod. Lets build the script. Lets declare our variables.
$NextCloudURL = "https://cloud.bolding.us/"
$ShareID = "oWHeW4dfWnxwXXX"
$SharePassword = "I'mAnAwesomePasswordDon'tYoVKnowThisRock$LikeCheesecake!"
Next, we need the item we are going to upload. We are going to use the Get-item command to grab that information.
$item = Get-Item c:\temp\upload\mycrazyideas.sqlite
Now the hard part. We need to create the header. This is where we will be placing the passwords and the type of information we are going to be accessing.
$Headers = @{
"Authorization" = "Basic "+[System.Convert]::ToBase64String([System.Text.Encoding]::UTF8.GetBytes("$($ShareID):$($SharePassword)"));
"X-Requested-With"="XMLHttpRequest";
}
The Authorization type is going to be basic. We are going to convert the shareid and the sharepassword into the UTF8 so our nextcloud can understand it. We want all that as a base 64. So we create the string with “$($ShareID):$($SharePassword)” and push that into our System.Text.Encoding UTF8. Using the method GetByes. All that is then put into the System.Convert base of 64 string, aka password.
Next, we tell the site what we are requesting, we are requesting the XML HTTP request. Next, we will create the URL that will be used by the rest method.
$URLBuild = "$($NextcloudUrl)/public.php/webdav/$($Item.Name)"
This is building a string for our URL for the rest method. It will look something like this:
- https://cloud.bolding.us/public.php/webdav/mycrazyideas.sqlite
Now we have the Header that will be needed for our rest method. We have the URL. now we need the method and the file. We do the file by using the -InFile and select the full name of the file $Item.Fullname. The method will be PUT as we are putting something somewhere.
Invoke-RestMethod -Uri $URLBuild -InFile $Item.Fullname -Headers $Headers -Method Put
Now it’s time to put it all together into a workable function.
Script
function Invoke-SHDUploadToNextCloud {
[cmdletbinding()]
param (
[string]$NextCloudURL,
[string]$ShareID,
[string]$SharePassword,
[string]$ItemPath
)
$item = Get-Item $ItemPath
$Headers = @{
"Authorization" = "Basic "+[System.Convert]::ToBase64String([System.Text.Encoding]::UTF8.GetBytes("$($ShareID):$($SharePassword)"));
"X-Requested-With"="XMLHttpRequest";
}
$URLBuild = "$($NextcloudUrl)/public.php/webdav/$($Item.Name)"
Invoke-RestMethod -Uri $URLBuild -InFile $Item.Fullname -Headers $Headers -Method Put
}