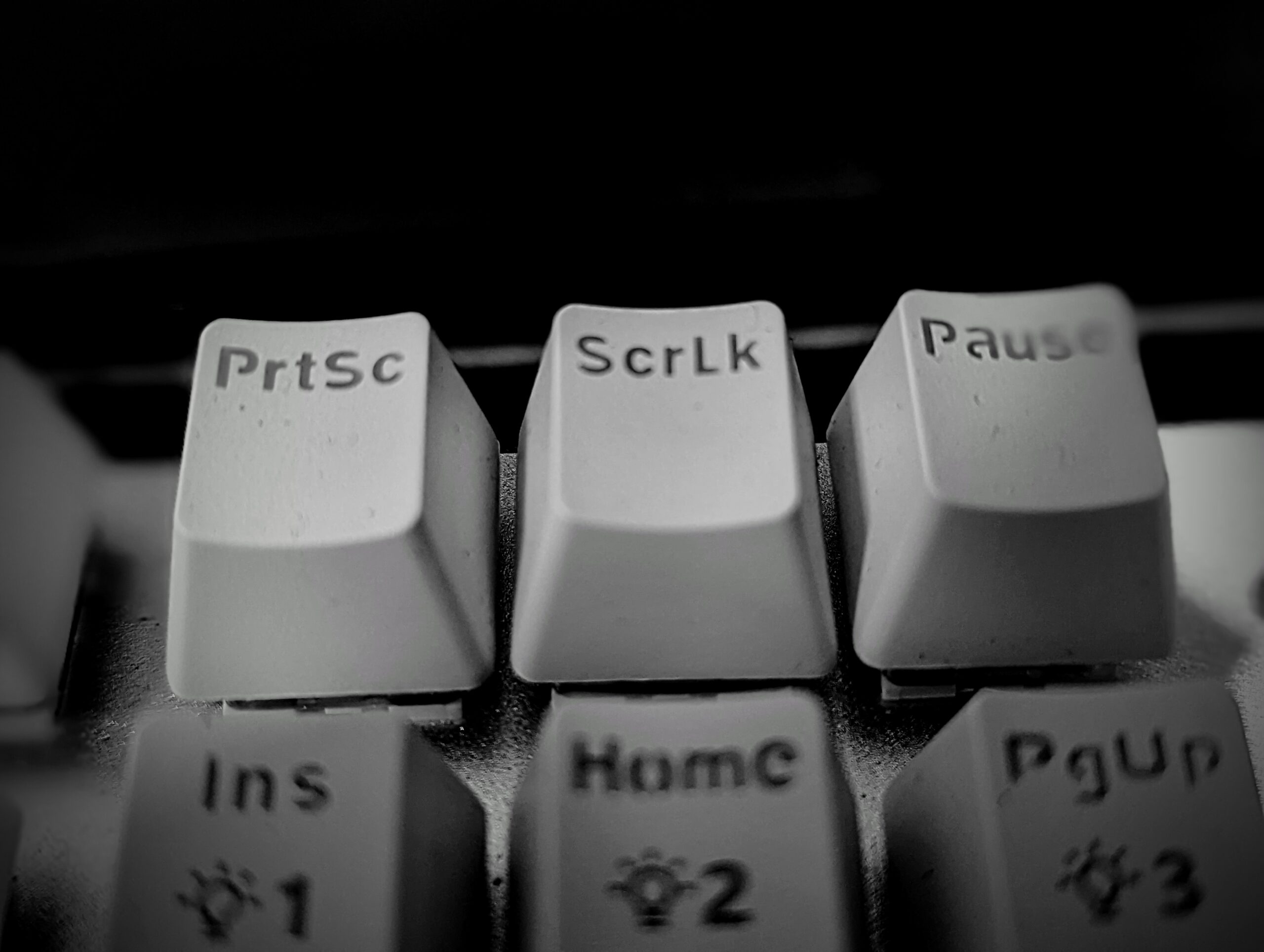
Wait for service to appear – PowerShell
This past week I had to install a piece of software that took 30 minutes to install. The software had multiple levels of processes that made the -wait feature completely useless. The best way to know the software was installed is to detect the service names. Thus you have to wait for service to appear. The fun part is depending on the windows 10 version, it would install upwards of 11 services.
Thankfully it followed a set pattern and the first 7 services were the only ones that we needed to watch for. Another wrinkle in this installer’s process is the computer couldn’t go to sleep or the screen couldn’t lock. This wrinkle had me think outside of the box a bit. I came up with two ideas. The first idea was to set the power configuration was set to never sleep and the screen not to lock. This would require exporting the config and importing. This was a lot of code, but doable. The second idea would tell the computer to click the scroll lock button over and over again. Guess which one I took? Yep, the scroll lock. Parsing random strings is fun and all, but can be accident-prone. Just to be fun about it, I decided to make things a little random with get-random. This way the system wouldn’t flag it as a virus.
The Script – Wait for service to appear
$WShell = New-Object -Com "Wscript.Shell"
$A = 0
Do {
$RandomNumber1 = Get-Random -Minimum 1 -Maximum 20
$RandomNumber2 = Get-Random -Minimum $RandomNumber1 -Maximum ($RandomNumber1 + 25)
$WShell.SendKeys("{SCROLLLOCK}")
Start-Sleep -Seconds $RandomNumber2
$A = $A + $RandomNumber2
} until ((Get-Service -Name "frog*").count -ge 7)
write-host "The system took around $A seconds to complete"
The Breakdown
The core of this code is a simple Do Until loop. The loop executes at least one time. Then it evaluates to see if it needs to execute again. We have a while and Until. While basically means while this is happening do this. The until says, keep doing it man until this is met. Here we are using a Do Until. The first step is to create the windows script shell. AKA wscript.shell. This allows us to send key commands and such. Next, we create a 0 variable to keep up with the time. Because I like seeing the time.
We enter the do loop… Dum Dum Do? We start off by making a random number between 1 and 20. We place that into a variable. Then we start another random number. The minimum is the last random number and the maximum is the last random number plus 25. Thus the maximum time is 45 seconds.
Next, we send the scroll lock key. using the Wscript.shell sendkeys. Then we sleep for a random time. We add that information into the variable. Then we come to the Until. We ask to see if the service is there with Get-service. Our services all start with frog. Thus we use the -name “frog*”. We get the count will be greater than 7. If it is greater than 7, then we exit the loop and tell the user how long it took to complete.
The fun part about this is you can set the service to something that will never exist and the unit will keep running until you stop it. This will keep your computer from locking. If your company doesn’t have good monitoring software, like most small businesses, then it will go unnoticed.
Conclusion
And that’s how you Wait for service to appear. It’s not glamorous, but PowerShell does help the process get along. This code’s main goal is to keep the computer awake for at least 30 minutes. Always remember, that great code comes with great ability.
More links: