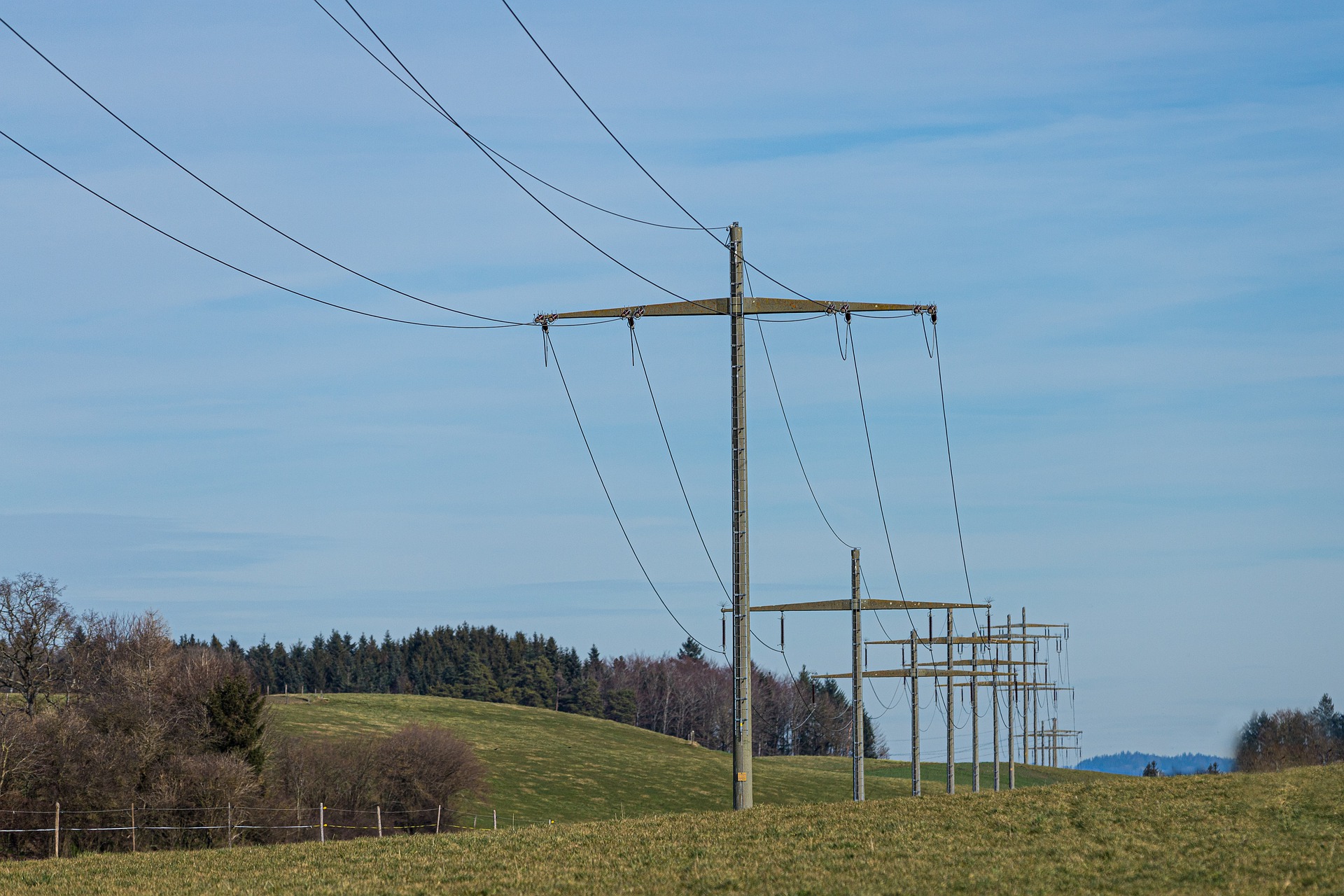
Test Microsoft Service Connections
This past week I have had multiple terminals up with different clients on different terminals connected to different Microsoft services. I quickly realized that I needed to know if I was already connected or not to each Microsoft service. I knew that Get-PPSession was a key to this, but what surprised me was azure and msols didn’t have a PPSession to review. I use 4 different connections on a daily basis. The Msol, Exchange Online, Compliance, and Azure AD. Here is how you can quickly test each service to see if it’s connected.
Msol Service
With Msol we want to test a command to see if it works. We output that command to null. The command I found to be the quickest for this is Get-MsolDomain. Once we run the command we check to see how successful that command was with $?. I like to put things into arrays for future use. So, I dropped it into a $Results and then present those display those results.
Get-MsolDomain -Erroraction SilentlyContinue | out-null
$Results = $?
$Results
Exchange Online
With Exchange online, we can use the Get-PSSession. We search the connection uri for outlook.office365. We also look at the state to be opened. We then ask if how many sessions there are. if it’s greater than 0, then we are good to go. Believe it or not, this is a one-liner.
(Get-PSSession | Where-object { ($_.ConnectionURI -like "*outlook.office365.com*") -and ($_.State -like "Opened")}).count -gt 0
Compliance
With Compliance, it’s similar to the exchange online. We are using the Get-PSSession again. This time we are looking for the word compliance and the state open as well. Once again, this can be a one-liner.
(Get-PSSession | where-object { ($_.ConnectionUri -like "*compliance*") -and ($_.State -like "Opened") } ).count -gt 0
Azure AD
Finally, we come to the Azure AD, Just like the Msol, we have to check it using a command. I have seen people use the Get-AzureADTenantDetail commandlet. So we will use that commandlet and pipe it into out-null. Then we confirm if it worked with the $? command.
Get-AzureADTenantDetail -ErrorAction SilentlyContinue | out-null
$Results = $?
$Results
The Function
Let’s combine it together into a function.
function Test-SHDo365ServiceConnection {
<#
.SYNOPSIS
Tests to see if you are connected to verious of services.
.DESCRIPTION
Tests to see if you are connected to Microsoft Online Services, Exhcange Online, Complience Center, and Azure AD.
.PARAMETER MsolService
[switch] - Connects to Microsoft Online Services
.PARAMETER ExchangeOnline
[switch] - Connects to Exchange Online
.PARAMETER Complience
[switch] - Connects to complience services
.PARAMETER AzureAD
[switch] - Connects to azure AD
.EXAMPLE
PS> Test-SHDo365ServiceConnection -MsolService
Gives a trur or False statement on weither connected or not.
.OUTPUTS
[pscustomobject]
.NOTES
Author: David Bolding
.LINK
https://github.com/rndadhdman/PS_Super_Helpdesk
#>
[cmdletbinding()]
param (
[switch]$MsolService,
[switch]$ExchangeOnline,
[Switch]$Complience,
[switch]$AzureAD
)
if ($MsolService) {
Get-MsolDomain -Erroraction SilentlyContinue | out-null; $Results = $?
[pscustomobject]@{
Service = "MsolService"
Connected = $Results
}
}
if ($ExchangeOnline) {
$Results = (Get-PSSession | Where-object { ($_.ConnectionUri -like "*outlook.office365.com*") -and ($_.State -like "Opened")}).count -gt 0
[pscustomobject]@{
Service = "ExchangeOnline"
Connected = $Results
}
}
if ($Complience) {
$Results = (Get-PSSession | where-object { ($_.ConnectionUri -like "*compliance*") -and ($_.State -like "Opened") } ).count -gt 0
[pscustomobject]@{
Service = "Complience"
Connected = $Results
}
}
if ($AzureAD) {
Get-AzureADTenantDetail -ErrorAction SilentlyContinue | out-null; $Results = $?
[pscustomobject]@{
Service = "AzureAD"
Connected = $Results
}
}
} #Review