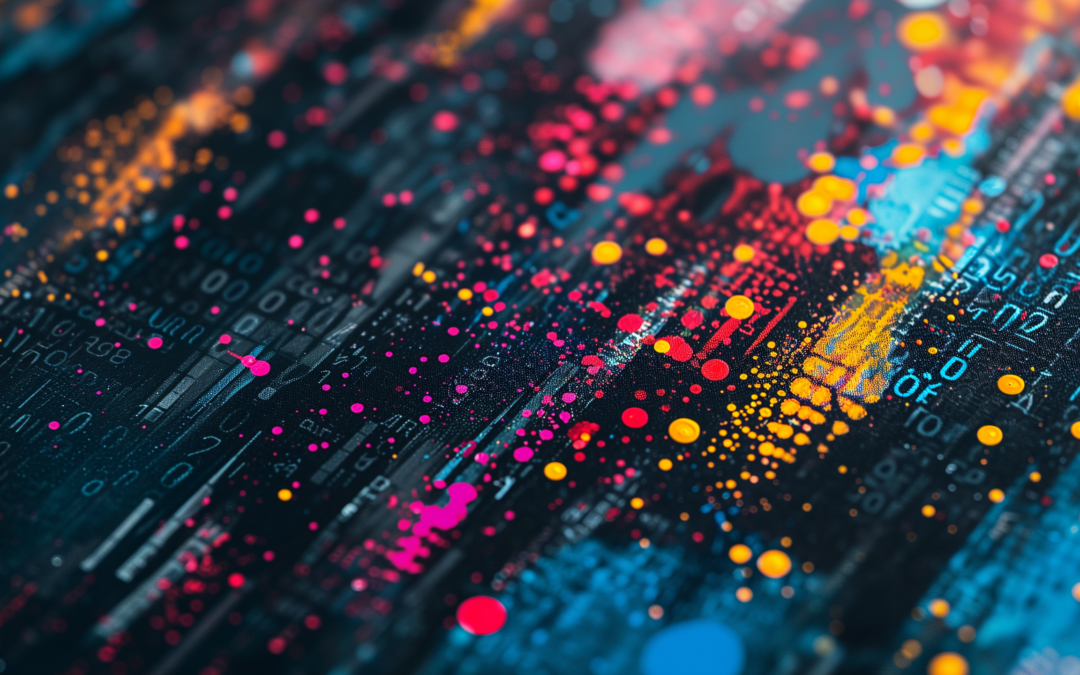
Building Parameters for Commands
One of my favorite things with powershell is building out splat parameters for commands through the main parameter set. Today we are going to go over how that is done. We are going to do this through the Get-childitem and get-ACL. These are some mighty commands and they can help you find permission gaps quickly and easily. Let us Building Parameters for Commands together.
The Script
Function Get-ACLInfos {
[cmdletbinding()]
param (
[string]$FilePath,
[switch]$Recurse,
[switch]$Directory,
[switch]$File,
[string]$Filter,
[string]$Username
)
begin {
if (!(Test-Path -Path $FilePath)) {end}
$Param = @{
Path = $FilePath
}
if ($Recurse) {$Param | Add-Member -MemberType NoteProperty -Name "Recurse" -Value $true}
if ($Directory) {$Param | Add-Member -MemberType NoteProperty -Name "Directory" -Value $true}
if ($File) {$Param | Add-Member -MemberType NoteProperty -Name "File" -Value $true}
if ($PSBoundParameters.ContainsKey($Filter)) {$Param | Add-Member -MemberType NoteProperty -Name "Filter" -Value "$Filter"}
}
process {
$Items = Get-ChildItem @Param
$ACLinfo = foreach ($item in $Items) {
$ACLs = (Get-Acl -Path $item.FullName).access
foreach ($ACL in $ACLs) {
[pscustomobject][ordered]@{
Path = $item.FullName
FileSystemRight = $ACL.FileSystemRights
AccessControlType = $ACL.AccessControlType
IdentityReference = $ACL.IdentityReference
IsInherited = $ACL.IsInherited
InheritanceFlags = $ACL.InheritanceFlags
PropagationFlags = $ACL.PropagationFlags
}
}
}
}
end {
if ($PSBoundParameters.ContainsKey('Username')) {
$ACLinfo | Where-Object {$_.IdentityReference -contains $Username}
} else {
$ACLinfo
}
}
}
Building Parameters for Commands
This script is simple, it allows you to grab a directory’s ACL recursively. However, how it does it is kind of cool. Get-Childitem has so many different options, but using it within a function can take some of that power away. So, by passing the parameters that we want at the top level allows us to give that power back to the get-childitem. However, this could lead to a lot of if statements. Instead, we are going to build a splat parameter for our commands. I have covered splats in a previous blog post, but I wanted to point them out to express how much power they do have.
The Power of Splat
Splatting is a method in which you can build a parameter set for a command. The amazing part of splatting is the splat is a powershell object. This means you can add to it after the initial splat is started. To start a splat all one has to do is declare a variable with an object attached. The variable is declared with the $ and the object is a simple at symbol followed by curly brackets with an equal sign nestled in the middle. We can use objects anywhere in powershell. A custom PS object is the same way. If you wanted to, you can declare the object and give it an order with [pscustomobject][order]. However, that’s not always the best option as orders cause issue if things are not in that order later down the road.
$Param = @{}
At this point the powershell object is empty. From here we can start building parameters for our object. To do that we are going to use the add-member command. In our example, we are working with true and false statements. Our function parameters are mostly switches. I threw in some strings as well to give you examples of how to build with a string. The first check is to see if we have a recursive. This is simple to do. We ask if the recurse is true. Then we add the member.
$Param = @{
Path = $FilePath
}
if ($Recurse) {$Param | Add-Member -MemberType NoteProperty -Name "Recurse" -Value $true}
Building the Splat
The add-member starts us down the path of building our splat. The Add-member can give us a lot of different options on which way we want to add things. Here, we need to add the recurse flag to get-childitem. This is a note property inside the command. The best way I see note properties is a name with a value. That value can be null. Here we are adding the “Recurse” name and giving it a value of the boolean true. Thus, when we drop the splat into the command, the command will see a flag of recurse. We do this same method with the rest. Directory is a flag, and so is file.
Unlike the last three, the filter parameter is a string. We are going to use the same method using the note property. We want to give the name as a filter and the value will be our value from our command line. The difference here is we want to place that filter in a string. The next part of the filter is how we test the filter. Instead of doing a simple check to see if the value is true, we need to check the parameters. This is done through the value $PSboundParameters. We want to see which keys the power shell bound parameters are holding. What this means is when you do get-command -something “bob” we want to know if something exists. Then we are going to use that’s something, aka bob.
$Param = @{
Path = $FilePath
}
if ($Recurse) {$Param | Add-Member -MemberType NoteProperty -Name "Recurse" -Value $true}
if ($Directory) {$Param | Add-Member -MemberType NoteProperty -Name "Directory" -Value $true}
if ($File) {$Param | Add-Member -MemberType NoteProperty -Name "File" -Value $true}
if ($PSBoundParameters.ContainsKey($Filter)) {$Param | Add-Member -MemberType NoteProperty -Name "Filter" -Value "$Filter"}
Finishing the Splat
The next step is to use the splat. Which is very easy to do. To use a splat, instead of using the dollar sign, all you need to do is use the at symbol and the parameter. The Command should not have any other flags set as it all lives inside the splat. Building a parameter splat is super easy and makes life easier in the long run.
$Items = Get-ChildItem @Param
What can we learn as a person from splatting
As we go through our lives, we are a representation of a splat. We start off with a few things and over time we add and remove aspects from ourselves. Others add and remove aspects of us as well. Growing up in school, teacher pours so much of their selves into their students. As we get older, we have to do the same for ourselves. We have to add the note properties to our lives, or we will always stay the same. Today you add-member -membertype noteproperty “Splatting” -value “dang it’s cool” to yourself. Unlike a computer though, we have to practice to bring it close to ourselves. We have to conceptualize the idea.
As you go through life, you have to depend on your own splat. Not enjoying that splat, means you have to work on it. It takes action to do so. The thing that stops most people from taking that action is fear of the unknown or fear of dealing with the pain. As someone who has been working on himself for many years now, I can safely say, it’s ok not to be ok. It’s ok not to enjoy parts of your splat, but overall, your splat is who you are. So go out into this world and put your @ on things. Change up that splat if you don’t like parts of it. Just enjoy being yourself.